React当表单完成选择后onChange监听当选择完成后查询出对应数据用函数组件ts
时间: 2023-06-12 13:04:49 浏览: 53
首先,你需要在函数组件中定义一个 `useState` 钩子来存储表单选择的值。当表单选择完成时,`onChange` 监听器将更新该状态值。接下来,在 `useEffect` 钩子中使用该状态值来查询对应的数据,然后将查询结果保存在另一个 `useState` 钩子中。最后,在组件中渲染这些数据。
以下是一个示例代码:
```tsx
import React, { useState, useEffect } from "react";
type OptionValue = string;
interface Option {
value: OptionValue;
label: string;
}
interface OptionData {
value: OptionValue;
data: any;
}
interface Props {
options: Option[];
fetchData: (value: OptionValue) => Promise<any>;
}
const FormWithSelect: React.FC<Props> = ({ options, fetchData }) => {
const [selectedOption, setSelectedOption] = useState<OptionValue | null>(null);
const [optionData, setOptionData] = useState<OptionData | null>(null);
useEffect(() => {
const fetchOptionData = async () => {
if (selectedOption) {
const data = await fetchData(selectedOption);
setOptionData({ value: selectedOption, data });
} else {
setOptionData(null);
}
};
fetchOptionData();
}, [selectedOption, fetchData]);
const handleOptionChange = (event: React.ChangeEvent<HTMLSelectElement>) => {
setSelectedOption(event.target.value);
};
return (
<>
<label htmlFor="select">Select an option:</label>
<select id="select" onChange={handleOptionChange}>
<option value="">-- Select an option --</option>
{options.map((option) => (
<option key={option.value} value={option.value}>
{option.label}
</option>
))}
</select>
{optionData && <div>{JSON.stringify(optionData.data)}</div>}
</>
);
};
export default FormWithSelect;
```
在上面的示例中,`FormWithSelect` 组件接受两个 props:`options` 和 `fetchData`。`options` 是一个选项数组,`fetchData` 是一个异步函数,用于根据选项值查询数据。
在组件中,我们使用 `useState` 钩子来存储选项值和查询结果。当选项值发生变化时,我们使用 `useEffect` 钩子来查询对应的数据,并将结果保存在 `optionData` 中。最后,我们在组件中渲染 `optionData` 中的数据。
注意,这只是一个简单的示例,你需要根据具体的需求来修改代码。
相关推荐
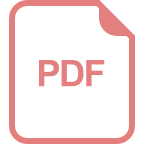
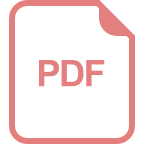
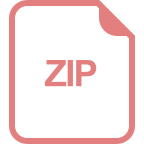
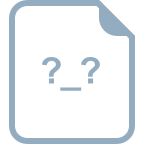
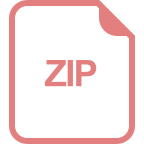
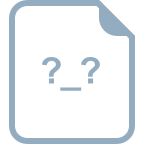
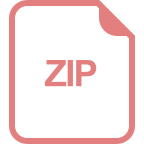
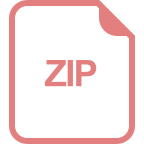
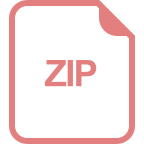
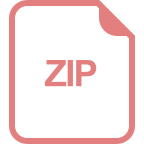
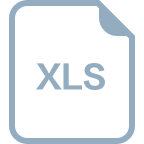
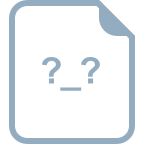