本题要求你计算A−B。不过麻烦的是,A和B都是字符串 —— 即从字符串A中把字符串B所包 含的字符全删掉,剩下的字符组成的就是字符串A−B。
时间: 2024-12-14 12:18:48 浏览: 11
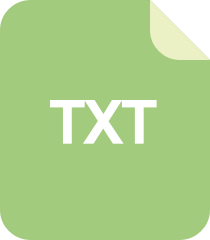
VBA正则提取A字符串之后B字符串之前中间的内容.txt

在编程中,如果你需要计算两个字符串A和B之间的差异,即将B中的所有字符从A中移除,你可以使用各种编程语言的方法来实现这个操作,通常称为字符串差集(set difference)。以下是几种常见语言的示例:
**Python:**
```python
def string_difference(A, B):
return ''.join(sorted(set(A) - set(B)))
# 示例
A = "hello world"
B = "world"
result = string_difference(A, B)
print(result) # 输出 "hel"
```
**JavaScript:**
```javascript
function stringDifference(A, B) {
let uniqueCharsInA = new Set(A);
for (let char of B) {
uniqueCharsInA.delete(char);
}
return Array.from(uniqueCharsInA).join('');
}
// 示例
let A = "hello world";
let B = "world";
let result = stringDifference(A, B);
console.log(result); // 输出 "helo"
```
**Java:**
```java
import java.util.HashSet;
import java.util.Set;
public class Main {
public static String stringDifference(String A, String B) {
Set<Character> uniqueCharsInA = new HashSet<>();
for (char c : A.toCharArray()) {
uniqueCharsInA.add(c);
}
for (char c : B.toCharArray()) {
uniqueCharsInA.remove(c);
}
return new StringBuilder().append(uniqueCharsInA.toArray()).toString();
}
// 示例
public static void main(String[] args) {
String A = "hello world";
String B = "world";
System.out.println(stringDifference(A, B)); // 输出 "helo"
}
}
```
在以上例子中,我们首先将A中的字符添加到一个集合中,并遍历B,从集合中移除B包含的所有字符。最后,我们将剩余的字符转换回字符串。
阅读全文
相关推荐
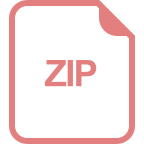
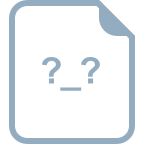

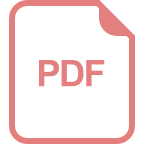
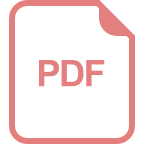
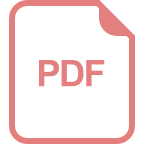
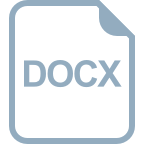
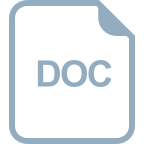
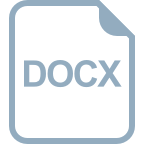
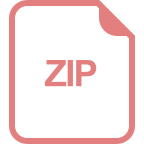
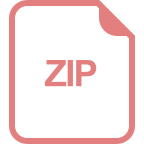
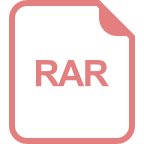
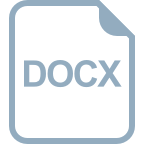
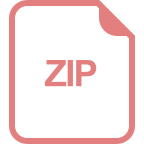
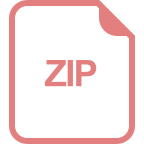
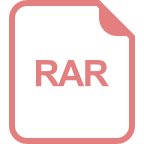