vc++实现凯撒密码
时间: 2024-10-10 16:17:31 浏览: 43
VC++(Visual C++)是一个集成开发环境,用于Microsoft Windows平台上的软件开发。要实现凯撒密码(Caesar Cipher),你可以创建一个简单的控制台应用程序。以下是步骤概览:
1. **新建项目**:打开Visual Studio,选择“新建”->“控制台应用”。
2. **添加源文件**:右键点击项目的解决方案资源管理器,选择“添加”->“新项”,然后选择“C++ File (.cpp)`。
3. **编写代码**:
- 在源文件中,首先包含必要的头文件,如`iostream`和`string`。
```cpp
#include <iostream>
#include <string>
```
- 定义函数,接收输入的明文、偏移量(例如,加密步长),并生成密文。可以使用for循环遍历字符串,并将每个字符替换为其字母表位置向后移动指定步长后的对应字符。
```cpp
std::string caesarCipher(const std::string& plaintext, int shift) {
std::string ciphertext;
for (char c : plaintext) {
if (isalpha(c)) {
c = static_cast<char>((c + shift - 'A') % 26 + 'A');
// 对小写字母也做同样的处理
if ('a' <= c && c <= 'z')
c = static_cast<char>((c + shift - 'a') % 26 + 'a');
}
ciphertext += c;
}
return ciphertext;
}
```
- 主程序部分,获取用户输入的明文和偏移量,调用上面定义的函数进行加密,并显示结果。
```cpp
int main() {
std::string input;
int shift;
std::cout << "Enter plain text: ";
getline(std::cin, input);
std::cout << "Enter shift value: ";
std::cin >> shift;
std::string encrypted = caesarCipher(input, shift);
std::cout << "Encrypted message: " << encrypted << std::endl;
return 0;
}
```
4. **运行程序**:编译并运行这个程序,按照提示输入明文和偏移量,即可看到加密的结果。
阅读全文
相关推荐
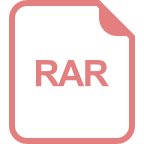
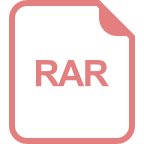
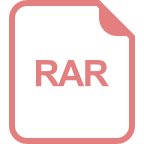

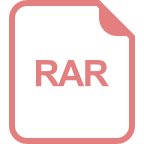
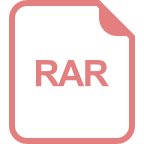
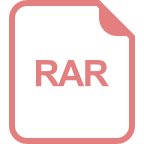
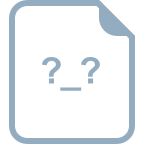
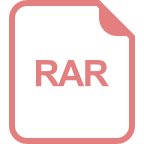
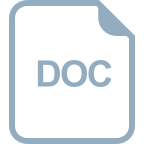
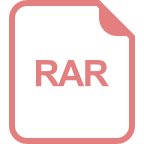
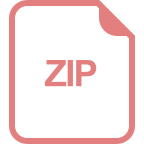
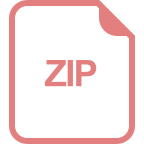
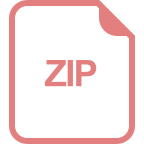
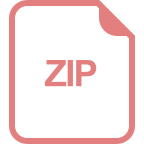
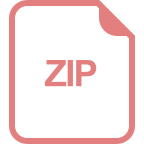