C语音字符串中查找字符
时间: 2024-09-27 19:17:34 浏览: 28
在C语言中,你可以使用`strstr()`函数来在一个字符串中查找另一个字符串。这个函数的原型是`char *strstr(const char *haystack, const char *needle)`,它会在`haystack`(主字符串)中搜索`needle`(子字符串)是否作为连续的字符序列存在,并返回第一次找到`needle`的指针,如果没找到则返回`NULL`。
例如:
```c
#include <string.h>
char str[] = "Hello, World!";
const char* found = strstr(str, "World");
if (found) {
printf("Substring found at position %ld\n", (long)(found - str));
} else {
printf("Substring not found.\n");
}
```
在这个例子中,`strstr()`会找到"World"并打印出它的起始位置。如果想查找的子串不存在于主串中,程序将输出"Substring not found."。
相关问题
c语音字符串删除
你可以使用C语言中的字符串函数来删除字符串中的字符。以下是一个简单的示例代码,可以删除一个字符串中的指定字符。
```c
#include <stdio.h>
#include <string.h>
int main()
{
char str[100], ch;
int i, j;
printf("Enter a string: ");
gets(str);
printf("Enter the character to delete: ");
scanf("%c", &ch);
for(i = 0; str[i] != '\0'; i++)
{
if(str[i] == ch)
{
for(j = i; str[j] != '\0'; j++)
{
str[j] = str[j + 1];
}
i--;
}
}
printf("Resultant string: %s", str);
return 0;
}
```
该程序从标准输入中读取一个字符串和一个字符,然后使用两个嵌套的循环来查找和删除该字符。最后,程序输出结果字符串。注意,这里使用了`gets()`函数,但它已经不再安全,可以使用`fgets()`函数代替。
用c语音在字符串1中删除字符串2
好的,我可以帮你解决这个问题。这里我提供一种简单的方法来实现这个功能,你可以参考下面的代码:
```c
#include <stdio.h>
#include <string.h>
void delete_str(char *str1, const char *str2) {
int len1 = strlen(str1);
int len2 = strlen(str2);
int i, j, k;
for (i = 0; i < len1 - len2 + 1; i++) {
for (j = 0, k = i; j < len2 && str1[k] == str2[j]; j++, k++);
if (j == len2) {
for (j = i, k = i + len2; k < len1; j++, k++) {
str1[j] = str1[k];
}
str1[j] = '\0';
i--; //继续从当前位置往后查找
}
}
}
int main() {
char str1[] = "abc123def456";
char str2[] = "def";
printf("Before: %s\n", str1);
delete_str(str1, str2);
printf("After: %s\n", str1);
return 0;
}
```
在这个代码中,我们定义了一个 `delete_str` 函数来实现在字符串1中删除字符串2的功能。具体实现方法是遍历整个字符串1,在每个位置上查找是否存在与字符串2相等的子串。如果找到了,则将该子串从字符串1中删除。
在主函数中,我们定义了两个字符串并调用 `delete_str` 函数来删除字符串2。最后输出结果,你可以在自己的环境中运行一下看看效果。
阅读全文
相关推荐
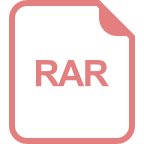
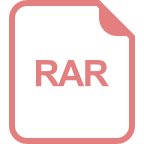
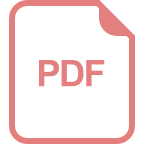
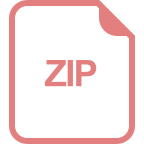
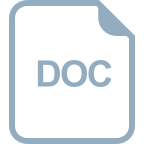
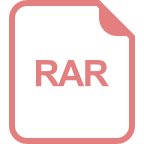
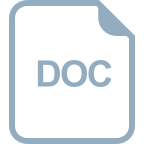
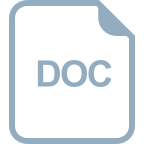
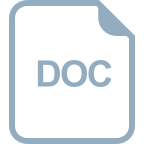
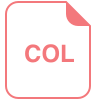
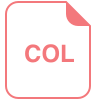
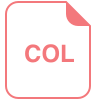
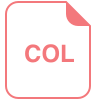
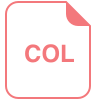
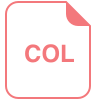
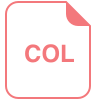
