C# winfrom程序中 生成1-255之间的随机数 如何把随机数转为三原色 瀑布图显示 第三方库选用
时间: 2024-11-09 21:24:48 浏览: 6
在C# WinForms程序中生成1-255之间的随机数并将其转换为三原色(RGB),然后使用瀑布图显示,可以使用第三方库如OxyPlot。以下是一个简单的示例代码,展示如何实现这一功能:
1. 首先,确保你已经安装了OxyPlot库。你可以通过NuGet包管理器安装它:
```
Install-Package OxyPlot.WindowsForms
```
2. 然后,在你的WinForms项目中添加一个`PlotView`控件到你的表单上。
3. 编写以下代码来生成随机数并绘制瀑布图:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using OxyPlot;
using OxyPlot.Series;
using OxyPlot.Axes;
namespace RandomColorWaterfall
{
public partial class Form1 : Form
{
private PlotView plotView;
private LineSeries lineSeries;
private Random random;
public Form1()
{
InitializeComponent();
InitializePlot();
random = new Random();
}
private void InitializePlot()
{
plotView = new PlotView
{
Dock = DockStyle.Fill,
Location = new System.Drawing.Point(0, 0),
Name = "plotView",
Size = new System.Drawing.Size(800, 600),
TabIndex = 0,
Text = "plotView"
};
this.Controls.Add(plotView);
var model = new PlotModel { Title = "Random Color Waterfall" };
lineSeries = new LineSeries
{
MarkerType = MarkerType.Circle,
MarkerSize = 4,
MarkerStroke = OxyColors.Black,
MarkerFill = OxyColors.White,
StrokeThickness = 1,
CanTrackerInterpolatePoints = false
};
model.Series.Add(lineSeries);
model.Axes.Add(new LinearAxis { Position = AxisPosition.Left, Minimum = 0, Maximum = 255, Title = "Value" });
model.Axes.Add(new CategoryAxis { Position = AxisPosition.Bottom, Title = "Index" });
plotView.Model = model;
}
private void UpdatePlot()
{
int value = random.Next(1, 256); // Generate a random number between 1 and 255
Color color = ColorFromTemperature(value); // Convert the number to a color
lineSeries.Points.Add(new DataPoint(lineSeries.Points.Count + 1, value) { Color = OxyColor.FromRgb(color.R, color.G, color.B) });
plotView.InvalidatePlot(true);
}
private Color ColorFromTemperature(int temp)
{
// Simple mapping from temperature (1-255) to an RGB color
byte r = (byte)(temp % 256);
byte g = (byte)((temp * 2) % 256);
byte b = (byte)((temp * 3) % 256);
return Color.FromArgb(r, g, b);
}
private void timer_Tick(object sender, EventArgs e)
{
UpdatePlot();
}
}
}
```
在这个示例中:
- `InitializePlot`方法初始化了OxyPlot的图表和系列。
- `UpdatePlot`方法生成一个1到255之间的随机数,并将其转换为颜色,然后将该点添加到瀑布图中。
- `ColorFromTemperature`方法将随机数映射到RGB颜色。
- `timer_Tick`方法定期调用`UpdatePlot`以更新图表。你可以在表单的构造函数中设置一个定时器来触发这个方法。
请确保你在表单设计器中添加了一个定时器控件,并将其`Tick`事件连接到`timer_Tick`方法。这样,每隔一段时间就会生成一个新的随机数并更新图表。
阅读全文
相关推荐
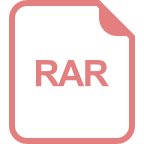
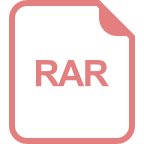
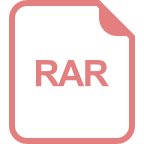

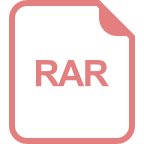
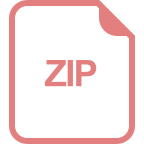
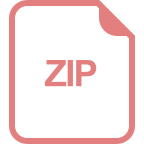
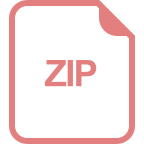
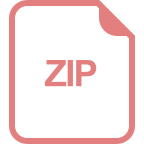
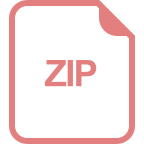
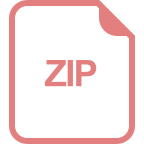
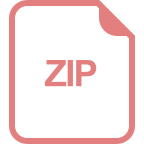
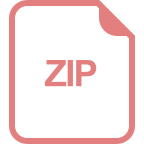