如何使用Java编程语言将服务器A目录下的文件移动到服务器B的指定路径?
时间: 2024-11-04 13:11:03 浏览: 6
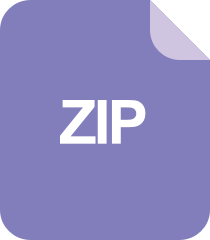
java上传文件到linux服务器,操作linux服务器上文件,下载linux服务器文件,删除linux服务器文件
在Java中,你可以使用`java.nio.file`包的类来实现文件的复制或移动(通常通过`Files.copy()`或`Files.move()`方法)。这里是一个基本的示例,展示如何从服务器A的一个目录下复制文件到服务器B的指定位置:
```java
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public void copyFileFromServerAToServerB(String serverAPath, String serverBDestinationPath) {
try {
// 获取服务器A的文件路径
Path sourcePath = Paths.get(serverAPath);
// 检查源文件是否存在
if (!Files.exists(sourcePath)) {
System.out.println("Source file not found at " + serverAPath);
return;
}
// 服务器B的绝对路径
Path destinationPath = Paths.get(serverBDestinationPath);
// 如果服务器B的路径不存在,尝试创建
if (!Files.exists(destinationPath.getParent())) {
Files.createDirectories(destinationPath.getParent());
}
// 使用Files.copy()方法复制文件
Files.copy(sourcePath, destinationPath, StandardCopyOption.REPLACE_EXISTING);
System.out.println("File copied from " + serverAPath + " to " + serverBDestinationPath);
} catch (IOException e) {
System.err.println("Error copying file: " + e.getMessage());
}
}
```
要使用这个方法,你需要替换`serverAPath`为服务器A上要复制的文件的实际路径,以及`serverBDestinationPath`为你想要在服务器B上存放文件的确切位置。
阅读全文
相关推荐
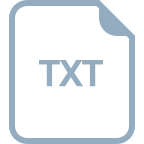
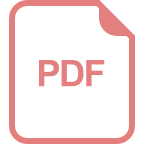
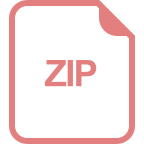
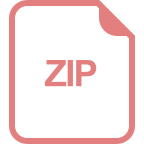
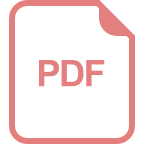
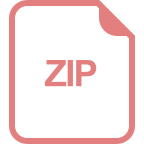
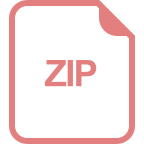
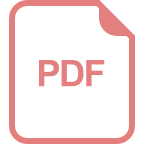
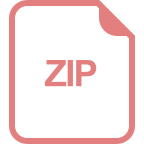
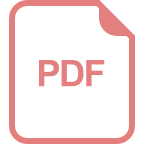
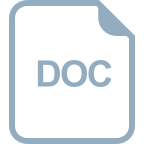
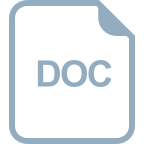
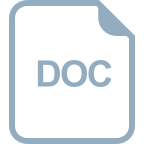
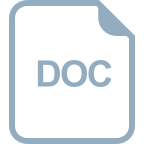
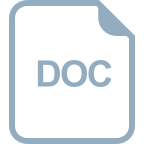

