Android Studio 中用kotlin语言写出完整代码(包含布局文件和具体代码),实现以下功能: 1.创建数据库person.db和表info(id,name,phone) 2.点击”添加“按钮可以往数据库perso.db中表info中添加一条记录,其中用户名为张三+随机数字,电话为8855+随机数字。 3.点击”查询所有“按钮把表中的信息显示到RecyclerView列表中。
时间: 2024-02-09 20:11:41 浏览: 176
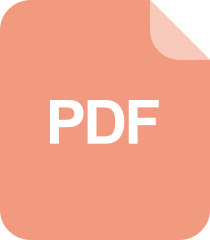
Android Studio使用Kotlin时,修改代码后运行不生效的解决方法
以下是使用Kotlin语言在Android Studio中实现该功能的完整代码,包括布局文件和具体代码:
布局文件 activity_main.xml:
```
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/btn_add"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="添加"
android:layout_marginTop="20dp"
android:layout_marginLeft="20dp"/>
<Button
android:id="@+id/btn_query_all"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="查询所有"
android:layout_marginTop="20dp"
android:layout_marginLeft="20dp"
android:layout_toRightOf="@id/btn_add"/>
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recycler_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@id/btn_add"
android:layout_marginTop="20dp"/>
</RelativeLayout>
```
数据模型类 Info.kt:
```
data class Info(val id: Int, val name: String, val phone: String)
```
数据库帮助类 DBHelper.kt:
```
class DBHelper(context: Context) : SQLiteOpenHelper(context, "person.db", null, 1) {
override fun onCreate(db: SQLiteDatabase?) {
db?.execSQL("create table info(_id integer primary key autoincrement, name text, phone text)")
}
override fun onUpgrade(db: SQLiteDatabase?, oldVersion: Int, newVersion: Int) {
}
}
```
主界面代码 MainActivity.kt:
```
class MainActivity : AppCompatActivity() {
private lateinit var dbHelper: DBHelper
private lateinit var infoList: MutableList<Info>
private lateinit var adapter: InfoAdapter
private lateinit var recyclerView: RecyclerView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
dbHelper = DBHelper(this)
findViewById<Button>(R.id.btn_add).setOnClickListener {
val name = "张三${Random.nextInt(100)}"
val phone = "8855${Random.nextInt(10000)}"
val db = dbHelper.writableDatabase
db.execSQL("insert into info(name, phone) values(?, ?)", arrayOf(name, phone))
db.close()
queryAll()
}
findViewById<Button>(R.id.btn_query_all).setOnClickListener {
queryAll()
}
recyclerView = findViewById(R.id.recycler_view)
val layoutManager = LinearLayoutManager(this)
recyclerView.layoutManager = layoutManager
infoList = mutableListOf()
adapter = InfoAdapter(infoList)
recyclerView.adapter = adapter
}
private fun queryAll() {
val db = dbHelper.writableDatabase
val cursor = db.query("info", null, null, null, null, null, null)
infoList.clear()
if (cursor.moveToFirst()) {
do {
val id = cursor.getInt(cursor.getColumnIndex("_id"))
val name = cursor.getString(cursor.getColumnIndex("name"))
val phone = cursor.getString(cursor.getColumnIndex("phone"))
infoList.add(Info(id, name, phone))
} while (cursor.moveToNext())
}
cursor.close()
db.close()
adapter.notifyDataSetChanged()
}
override fun onDestroy() {
super.onDestroy()
dbHelper.close()
}
}
class InfoAdapter(val infoList: List<Info>) :
RecyclerView.Adapter<InfoAdapter.ViewHolder>() {
inner class ViewHolder(view: View) : RecyclerView.ViewHolder(view) {
val nameView: TextView = view.findViewById(R.id.name)
val phoneView: TextView = view.findViewById(R.id.phone)
}
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context)
.inflate(R.layout.info_item, parent, false)
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
val info = infoList[position]
holder.nameView.text = info.name
holder.phoneView.text = info.phone
}
override fun getItemCount() = infoList.size
}
```
列表项布局文件 info_item.xml:
```
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="10dp">
<TextView
android:id="@+id/name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"/>
<TextView
android:id="@+id/phone"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:layout_marginLeft="10dp"/>
</LinearLayout>
```
阅读全文
相关推荐
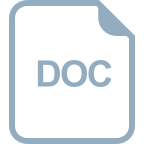
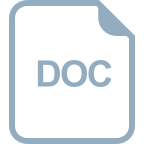
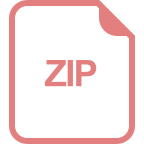
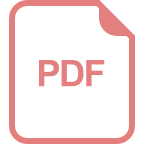
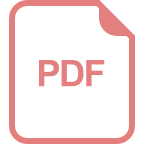
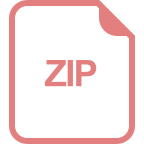
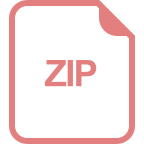
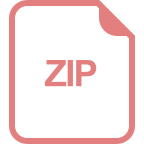
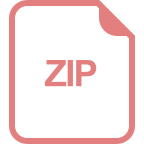
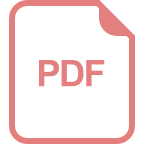
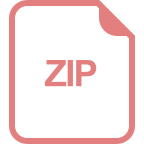
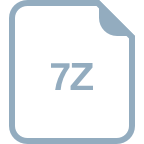
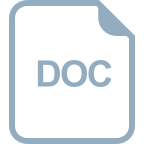



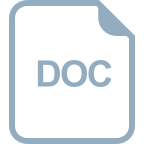
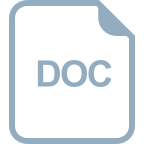