用python语言实现A星算法5*5迷宫寻路问题完整代码
时间: 2023-12-31 09:04:47 浏览: 62
好的,以下是用 Python 实现 A* 算法解决 5*5 迷宫寻路问题的完整代码:
```
import heapq
# 定义迷宫地图
maze_map = [
[1, 0, 1, 1, 1],
[1, 0, 1, 0, 1],
[1, 0, 1, 0, 1],
[1, 0, 1, 0, 0],
[1, 1, 1, 1, 1]
]
# 定义行走方向
directions = [(0, 1), (0, -1), (1, 0), (-1, 0)]
# 定义节点类
class Node:
def __init__(self, x, y, g, h, f, parent):
self.x = x
self.y = y
self.g = g
self.h = h
self.f = f
self.parent = parent
def __lt__(self, other):
return self.f < other.f
def __eq__(self, other):
return self.x == other.x and self.y == other.y
# 计算曼哈顿距离
def manhattan_distance(x1, y1, x2, y2):
return abs(x1 - x2) + abs(y1 - y2)
# A*算法求解迷宫
def find_path(start_x, start_y, end_x, end_y):
open_list = []
closed_list = []
start_node = Node(start_x, start_y, 0, manhattan_distance(start_x, start_y, end_x, end_y),
0 + manhattan_distance(start_x, start_y, end_x, end_y), None)
heapq.heappush(open_list, start_node)
while len(open_list) > 0:
current_node = heapq.heappop(open_list)
if current_node.x == end_x and current_node.y == end_y:
path = []
while current_node is not None:
path.append((current_node.x, current_node.y))
current_node = current_node.parent
return path[::-1]
closed_list.append(current_node)
for direction in directions:
next_x = current_node.x + direction[0]
next_y = current_node.y + direction[1]
if next_x < 0 or next_y < 0 or next_x >= len(maze_map) or next_y >= len(maze_map[0]):
continue
if maze_map[next_x][next_y] == 0:
continue
next_node_g = current_node.g + 1
next_node_h = manhattan_distance(next_x, next_y, end_x, end_y)
next_node_f = next_node_g + next_node_h
next_node = Node(next_x, next_y, next_node_g, next_node_h, next_node_f, current_node)
if next_node in closed_list:
continue
if next_node not in open_list:
heapq.heappush(open_list, next_node)
return None
# 测试代码
path = find_path(0, 0, 4, 4)
if path is None:
print("无法到达终点")
else:
print("找到路径:", path)
```
请注意,此代码仅用于演示如何使用 Python 实现 A* 算法解决 5*5 迷宫寻路问题,具体实现过程可能因应用场景不同而有所差异。
相关推荐
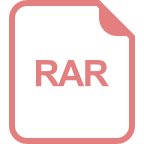













