vue界面和springboot后端分段传文件
时间: 2024-04-05 09:31:30 浏览: 204
您好,要实现Vue界面和Spring Boot后端分段传输文件,可以参考以下步骤:
1. Vue界面发送文件请求到Spring Boot后端,请求中包含文件名和文件大小等信息。
2. Spring Boot后端根据请求中的信息,将文件分成固定大小的若干个分段。
3. Spring Boot后端将每个分段依次返回给Vue界面,Vue界面接收到每个分段后,将其保存到本地。
4. 重复步骤3,直到Spring Boot后端将所有分段都返回给Vue界面,此时文件传输完成。
在Spring Boot后端中,可以使用RandomAccessFile类来实现文件的分段读取和发送。在Vue界面中,可以使用XMLHttpRequest对象来发送文件请求,并通过监听onprogress事件来实现分段接收文件数据。
除此之外,您还需要注意以下几点:
1. 在Spring Boot后端中,需要配置CORS(跨域资源共享)以允许Vue界面跨域请求后端服务。
2. 在Vue界面中,需要注意对接收到的每个分段数据进行合并,以便最终生成完整的文件。
希望以上信息对您有所帮助。
相关问题
vue界面和springboot后端分段传文件代码
以下是一个简单的Vue界面和Spring Boot后端分段传输文件的代码示例:
Vue界面代码:
```
<template>
<div>
<input type="file" @change="selectFile">
<button @click="uploadFile">上传文件</button>
</div>
</template>
<script>
export default {
data() {
return {
file: null,
fileSize: 0,
chunkSize: 1024 * 1024, // 每个分段大小为1MB
chunks: [],
uploadedChunks: []
}
},
methods: {
selectFile(event) {
this.file = event.target.files[0]
this.fileSize = this.file.size
this.chunks = this.splitFileIntoChunks()
this.uploadedChunks = []
},
splitFileIntoChunks() {
const chunks = []
let chunkIndex = 0
let offset = 0
while (offset < this.fileSize) {
const chunk = this.file.slice(offset, offset + this.chunkSize)
chunks.push({
index: chunkIndex,
file: chunk,
uploaded: false
})
offset += this.chunkSize
chunkIndex++
}
return chunks
},
async uploadFile() {
for (let i = 0; i < this.chunks.length; i++) {
const chunk = this.chunks[i]
if (!chunk.uploaded) {
await this.uploadChunk(chunk)
}
}
},
uploadChunk(chunk) {
return new Promise((resolve, reject) => {
const formData = new FormData()
formData.append('file', chunk.file)
formData.append('index', chunk.index)
formData.append('total', this.chunks.length)
const xhr = new XMLHttpRequest()
xhr.open('POST', 'http://localhost:8080/upload')
xhr.setRequestHeader('Content-Type', 'multipart/form-data')
xhr.onload = () => {
if (xhr.status === 200) {
chunk.uploaded = true
this.uploadedChunks.push(chunk)
resolve()
} else {
reject()
}
}
xhr.onerror = () => {
reject()
}
xhr.send(formData)
})
}
}
}
</script>
```
Spring Boot后端代码:
```
@RestController
public class FileController {
private static final Logger logger = LoggerFactory.getLogger(FileController.class);
@PostMapping(value = "/upload", consumes = MediaType.MULTIPART_FORM_DATA_VALUE)
public ResponseEntity<?> uploadFile(@RequestParam("file") MultipartFile file,
@RequestParam("index") int index,
@RequestParam("total") int total) {
try {
// 检查上传的文件是否为空
if (file.isEmpty()) {
return ResponseEntity.badRequest().body("上传的文件不能为空");
}
// 创建目录
File uploadDir = new File("upload");
if (!uploadDir.exists()) {
uploadDir.mkdir();
}
// 保存分段文件
File chunkFile = new File(uploadDir, index + ".part");
file.transferTo(chunkFile);
// 检查所有分段文件是否都已上传
if (index == total - 1) {
File outputFile = new File(uploadDir, file.getOriginalFilename());
FileOutputStream outputStream = new FileOutputStream(outputFile);
for (int i = 0; i < total; i++) {
File partFile = new File(uploadDir, i + ".part");
FileInputStream inputStream = new FileInputStream(partFile);
IOUtils.copy(inputStream, outputStream);
inputStream.close();
partFile.delete();
}
outputStream.close();
logger.info("文件 {} 上传成功", file.getOriginalFilename());
}
return ResponseEntity.ok().build();
} catch (IOException e) {
logger.error("文件上传失败", e);
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("文件上传失败");
}
}
}
```
以上代码仅供参考,具体实现还需要根据您的实际需求进行调整。
阅读全文
相关推荐
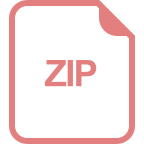
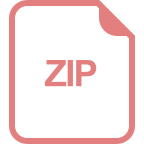
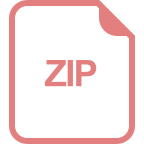
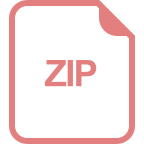
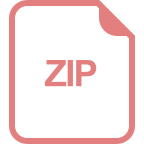
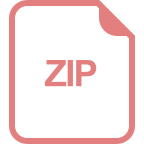
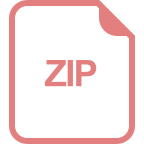
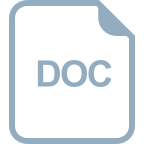
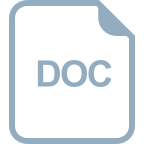
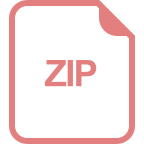
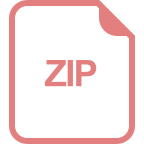
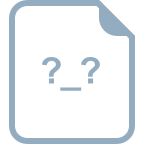
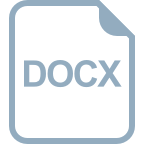