javascript编写的快速排序算法代码
时间: 2023-10-21 19:12:09 浏览: 40
以下是一个使用 JavaScript 编写的快速排序算法代码:
```javascript
function quickSort(arr) {
if (arr.length <= 1) {
return arr;
}
const pivot = arr[0];
const left = [];
const right = [];
for (let i = 1; i < arr.length; i++) {
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
return [...quickSort(left), pivot, ...quickSort(right)];
}
// 示例
const arr = [5, 3, 7, 1, 8, 2, 9, 4, 6];
console.log(quickSort(arr)); // 输出 [1, 2, 3, 4, 5, 6, 7, 8, 9]
```
该算法使用递归和分治的思想,将数组分为左右两部分,以第一个元素为基准,比它小的放在左边,比它大的放在右边,再对左右两部分分别递归进行快速排序,最后将排好序的数组合并返回。
相关问题
use javascript 生成快速排序
### 回答1:
以下是 JavaScript 生成快速排序的代码:
```
function quickSort(array) {
if (array.length <= 1) {
return array;
}
const pivot = array[0];
const left = [];
const right = [];
for (let i = 1; i < array.length; i++) {
if (array[i] < pivot) {
left.push(array[i]);
} else {
right.push(array[i]);
}
}
return quickSort(left).concat([pivot], quickSort(right));
}
```
使用方法:
```
const array = [9, 2, 6, 4, 3, 5, 1, 8, 7];
const result = quickSort(array);
console.log(result); // [ 1, 2, 3, 4, 5, 6, 7, 8, 9 ]
```
### 回答2:
快速排序是一种常用的排序算法,可以使用 JavaScript 来实现。
快速排序的基本思想是通过选择一个基准元素,将数组分为较小和较大两部分,再对这两部分分别进行快速排序,直到排序完成。
下面是用 JavaScript 实现快速排序的代码:
```javascript
function quickSort(arr) {
if (arr.length <= 1) {
return arr;
}
const pivotIndex = Math.floor(arr.length / 2);
const pivot = arr.splice(pivotIndex, 1)[0];
const left = [];
const right = [];
for (let i = 0; i < arr.length; i++) {
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
return quickSort(left).concat([pivot], quickSort(right));
}
const arr = [8, 3, 2, 9, 1, 7, 6, 4, 5];
const sortedArr = quickSort(arr);
console.log(sortedArr);
```
上述代码中,首先定义了一个名为 `quickSort` 的函数,它接受一个数组作为参数。如果数组长度小于等于 1,直接返回该数组。
接下来,选择一个基准元素,并从原数组中删除该元素。然后,依次遍历原数组中的元素,将小于基准元素的放入一个新数组 `left`,大于等于基准元素的放入另一个新数组 `right`。
最后,通过递归调用 `quickSort` 函数对 `left` 和 `right` 进行快速排序,并返回合并后的结果。
最后,我们使用一个示例数组 `[8, 3, 2, 9, 1, 7, 6, 4, 5]` 来测试这个快速排序算法,并将结果打印在控制台上。
运行以上代码,会输出排序后的数组 `[1, 2, 3, 4, 5, 6, 7, 8, 9]`。这就是通过 JavaScript 实现的快速排序算法。
### 回答3:
快速排序(Quick Sort)是一种常用的排序算法,利用分治策略将一个数组分成两个子数组,然后递归地排序两个子数组。在排序过程中,选择一个基准值,通过将比基准值小的元素移到基准值的左边,比基准值大的元素移到右边,实现排序。
下面是使用JavaScript编写的快速排序算法示例:
```javascript
// 定义快速排序函数
function quickSort(arr) {
// 判断数组长度小于等于1时直接返回
if (arr.length <= 1) {
return arr;
}
// 选择基准值,将数组分成两个子数组
let pivotIndex = Math.floor(arr.length / 2);
let pivot = arr.splice(pivotIndex, 1)[0];
let left = [];
let right = [];
// 遍历数组,比基准值小的元素放到left数组,比基准值大的元素放到right数组
for (let i = 0; i < arr.length; i++) {
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
// 递归地对左右子数组进行排序,并拼接返回结果
return quickSort(left).concat([pivot], quickSort(right));
}
// 测试快速排序算法
let arr = [5, 3, 8, 4, 2, 7, 1, 6];
console.log("排序前:" + arr);
let sortedArr = quickSort(arr);
console.log("排序后:" + sortedArr);
```
以上代码定义了一个名为`quickSort`的函数,实现了快速排序算法。在测试部分,定义了一个数组`arr`并打印排序前的数组,然后调用`quickSort`函数进行排序,并打印排序后的数组。
运行此代码,将会输出如下结果:
```
排序前:5,3,8,4,2,7,1,6
排序后:1,2,3,4,5,6,7,8
```
这说明快速排序算法正确地对数组进行了排序。
用JS写一个快速排序算法
### 回答1:
好的,我可以为您提供一个用JavaScript编写的快速排序算法。代码如下:
```
function quickSort(arr) {
if (arr.length <= 1) {
return arr;
}
const pivotIndex = Math.floor(arr.length / 2);
const pivot = arr[pivotIndex];
const left = [];
const right = [];
for (let i = 0; i < arr.length; i++) {
if (i === pivotIndex) {
continue;
}
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
return [...quickSort(left), pivot, ...quickSort(right)];
}
```
这个算法接受一个数组作为输入,然后通过递归地将数组分成较小的子数组并进行排序,最终返回排序好的数组。
### 回答2:
快速排序算法是一种常见的排序算法,它基于分治的思想。下面是用JavaScript实现快速排序的代码:
```
function quickSort(arr) {
if (arr.length <= 1) {
return arr;
}
const pivot = arr[Math.floor(arr.length / 2)];
const left = [];
const right = [];
for (let i = 0; i < arr.length; i++) {
if (i === Math.floor(arr.length / 2)) {
continue;
}
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
return [...quickSort(left), pivot, ...quickSort(right)];
}
// 示例
const arr = [5, 2, 8, 4, 9, 1, 3];
const sortedArr = quickSort(arr);
console.log(sortedArr); // 输出 [1, 2, 3, 4, 5, 8, 9]
```
这段代码首先判断了输入的数组长度是否小于等于1,如果是的话直接返回原数组,作为递归的终止条件。否则,取数组中间位置的元素作为基准值(pivot),并创建两个空数组用来存放左右两侧的元素。
然后,通过遍历数组中除了中间元素的其他元素,将小于基准值的元素放入左数组,大于等于基准值的元素放入右数组。
最后,通过递归调用quickSort函数对左右数组进行排序,并以 `[...quickSort(left), pivot, ...quickSort(right)]` 的形式返回排序后的数组。
使用示例中的数组 `[5, 2, 8, 4, 9, 1, 3]` 经过快速排序后得到 `[1, 2, 3, 4, 5, 8, 9]`。
快速排序算法的时间复杂度为 O(nlogn),是一种高效的排序算法。
### 回答3:
快速排序是一种常用的排序算法,它使用分治法来对数据集合进行排序。下面是用JavaScript语言编写的快速排序算法:
```javascript
function quickSort(arr) {
if (arr.length <= 1) {
return arr;
}
var pivotIndex = Math.floor(arr.length / 2);
var pivot = arr.splice(pivotIndex, 1)[0];
var left = [];
var right = [];
for (var i = 0; i < arr.length; i++) {
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
return quickSort(left).concat([pivot], quickSort(right));
}
// 测试快速排序算法
var array = [5, 2, 6, 3, 1, 4];
console.log(quickSort(array)); // 输出 [1, 2, 3, 4, 5, 6]
```
上述代码中,`quickSort`函数是递归函数,它将待排序的数组分成两部分,然后再对这两部分分别进行快速排序,最后将左侧部分、基准值和右侧部分拼接起来,得到排序后的数组。
快速排序的时间复杂度取决于分割点的选择,一般情况下平均时间复杂度为O(nlogn),最坏情况下的时间复杂度为O(n^2)。快速排序是一种原地排序算法,不需要额外的存储空间,所以空间复杂度为O(logn)。
相关推荐
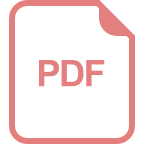
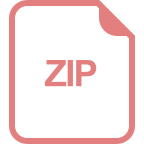
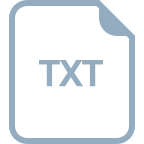












