遗传算法和cplex求解
时间: 2023-05-16 20:02:49 浏览: 506
遗传算法和cplex求解是常用的优化算法。由于问题的复杂度和不同问题之间的差异,选用不同的算法可以得到较好的结果。
遗传算法是一种基于生物进化方式的群体智能算法,通过模拟遗传发展过程迭代优化,逐步得到最优解。它对问题的初始解和搜索空间的定义较为自由,适用于非线性问题和无法求导的函数。
cplex是一种优化软件,可以求解复杂的线性规划和混合整数线性规划问题。cplex利用线性规划的理论和方法,在可行解和最优解之间寻求最优的解,具有较高的求解效率和精度,适用于大规模和精确求解的问题。
对于不同的问题,选择合适的算法可以提高求解效率和准确性。在考虑问题复杂度和求解精度的基础上,可以比较遗传算法和cplex求解的优缺点,从而选择最优的算法。
相关问题
matlab中cplex求解器算法原理
CPLEX是一种商业化的线性规划(LP)和整数规划(IP)求解器,它采用分支定界法(Branch-and-Bound)和割平面法(Cutting Plane)等方法对问题进行求解。
在求解LP问题时,CPLEX采用的是单纯形法(Simplex Method)和内点法(Interior Point Method)等方法。单纯形法是一种基于顶点的迭代算法,通过不断移动顶点来搜索最优解。内点法则是一种基于对偶问题的迭代算法,通过不断在可行域内移动中心点来搜索最优解。CPLEX根据问题的特性选择适合的方法进行求解。
在求解IP问题时,CPLEX采用的是分支定界法。该方法将问题分解成子问题,并通过约束条件对子问题进行削减。然后,对每个子问题进行求解,直到找到最优解或者确定问题无解。分支定界法通常需要进行割平面处理来进一步提高求解效率。
除此之外,CPLEX还具有很多高级功能,如多目标规划(MOP)、混合整数线性规划(MILP)和二次规划(QP)等。它还支持多种接口,如MATLAB接口和Python接口等,方便用户进行编程求解。
pyomo使用cplex求解器,怎么安装cplex求解器
要在Pyomo中使用CPLEX求解器,你需要先安装CPLEX软件,并配置Pyomo以使用CPLEX求解器。下面是安装CPLEX求解器的一般步骤:
1. 下载CPLEX软件:访问IBM的官方网站,下载适用于你的操作系统的CPLEX软件。请确保下载与你的操作系统和Python版本兼容的版本。
2. 安装CPLEX软件:按照官方文档提供的说明,运行安装程序,并按照向导中的步骤完成安装过程。确保选择适用于Python的安装选项。
3. 配置环境变量:在安装完成后,你需要将CPLEX的安装路径添加到系统的环境变量中。具体步骤取决于你使用的操作系统。在Windows上,你可以在“控制面板”中找到“系统”>“高级系统设置”>“环境变量”来进行配置。
4. 安装CPLEX Python API:CPLEX提供了Python API,允许Pyomo与CPLEX求解器进行交互。你可以使用pip命令来安装CPLEX Python API。在终端或命令提示符中运行以下命令:
```
pip install cplex
```
这将安装CPLEX Python API,并使其可用于Pyomo。
5. 配置Pyomo以使用CPLEX求解器:在Pyomo代码中,你需要指定使用CPLEX求解器。在创建Pyomo求解器对象时,可以指定使用CPLEX求解器。例如:
```python
from pyomo.environ import *
model = ConcreteModel()
# 定义模型和变量
# 创建求解器对象并指定CPLEX求解器
solver = SolverFactory('cplex')
# 求解模型
solver.solve(model)
```
请注意,如果你在安装CPLEX软件时选择了默认安装路径,Pyomo通常能够自动找到CPLEX求解器。
安装和配置CPLEX求解器可能会因操作系统和软件版本而有所不同。建议参考IBM的官方文档和支持资源,以获得关于安装和配置CPLEX求解器的详细说明。
希望这个回答对你有帮助!如果你还有其他问题,请随时提问。
相关推荐
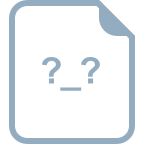












