用 c 语言实现,链表实现学生成绩管理系统
时间: 2024-12-15 18:31:06 浏览: 30
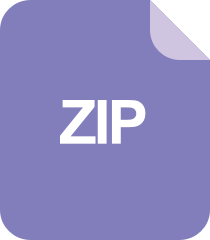
aa.zip_学生成绩管理系统 c语言_学生成绩链表

在C语言中,我们可以使用链表数据结构来实现一个简单的学生成绩管理系统。首先,我们需要创建一些基本的数据结构,如学生节点(Student Node)和成绩节点(Score Node),它们分别包含学生的ID、姓名和成绩等信息。以下是链表操作的一些关键步骤:
1. **定义数据结构**:
```c
typedef struct Student {
int id;
char name[50];
struct ScoreNode *scores; // 存储成绩的链表指针
} Student;
typedef struct ScoreNode {
int score;
struct ScoreNode *next;
} ScoreNode;
```
2. **初始化链表**:
- 对于每个学生,分配一个新的`Student`结构,并初始化其`scores`为NULL。
- 对于成绩链表,如果某门课程的成绩存在,则添加到对应学生的成绩链表中。
3. **插入新学生**:
```c
void addStudent(Student **students, int id, char* name) {
Student *newStudent = (Student*)malloc(sizeof(Student));
newStudent->id = id;
strcpy(newStudent->name, name);
newStudent->scores = NULL;
(*students)->next = newStudent;
*students = newStudent;
}
```
4. **插入成绩**:
```c
void addScore(Student *student, int course_id, int score) {
ScoreNode *newScore = (ScoreNode*)malloc(sizeof(ScoreNode));
newScore->score = score;
newScore->next = student->scores;
student->scores = newScore;
}
```
5. **查询成绩**:
```c
int getScore(Student *student, int course_id) {
for (ScoreNode *score = student->scores; score != NULL; score = score->next) {
if (score->course_id == course_id) {
return score-1; // 如果未找到,返回-1表示不存在该成绩
}
```
6. **删除元素**:
- 删除学生或成绩需要额外的逻辑,例如记录下一个节点并释放当前节点的内存。
阅读全文
相关推荐
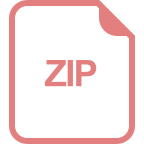
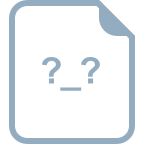
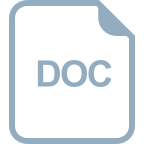
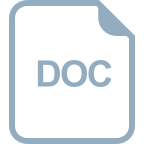
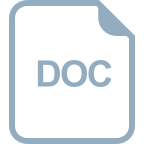
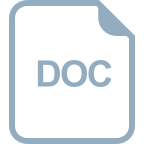

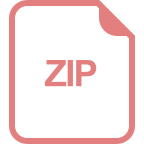
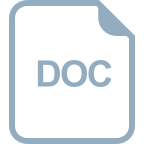
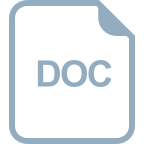
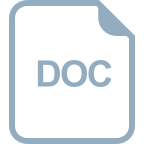
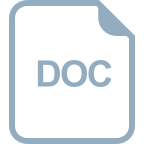
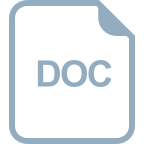

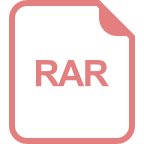
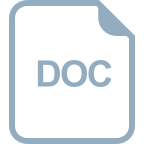
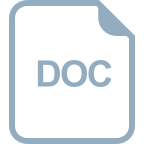