python中for循环详解
时间: 2023-12-03 21:39:47 浏览: 44
Python中的for循环可以用于遍历任何可迭代对象,例如列表、元组、字符串、字典等。for循环的语法结构为:`for 变量 in 可迭代对象:`,其中变量是在每次循环中被赋值的迭代元素,可迭代对象是需要遍历的对象。for循环的逻辑是在每次循环中,将可迭代对象中的下一个元素赋值给变量,然后执行循环体中的代码,直到可迭代对象中的所有元素都被遍历完毕。
以下是一些for循环的例子:
1. 遍历列表
```python
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
# 输出:
# apple
# banana
# cherry
```
2. 遍历元组
```python
numbers = (1, 2, 3)
for number in numbers:
print(number)
# 输出:
# 1
# 2
# 3
```
3. 遍历字符串
```python
string = "hello"
for char in string:
print(char)
# 输出:
# h
# e
# l
# l
# o
```
4. 遍历字典
```python
person = {"name": "Alice", "age": 25, "gender": "female"}
for key in person:
print(key, person[key])
# 输出:
# name Alice
# age 25
# gender female
```
5. 嵌套循环
```python
for i in range(1, 4):
for j in range(1, 3):
print(i, j)
# 输出:
# 1 1
# 1 2
# 2 1
# 2 2
# 3 1
# 3 2
```
6. 循环控制语句
```python
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
if fruit == "banana":
break
print(fruit)
# 输出:
# apple
for fruit in fruits:
if fruit == "banana":
continue
print(fruit)
# 输出:
# apple
# cherry
```
相关推荐
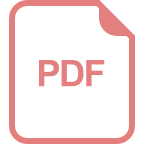
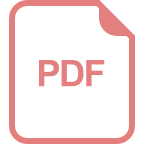
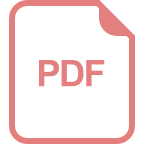
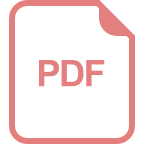
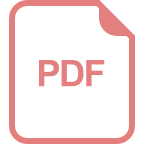
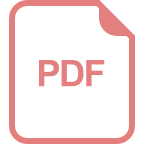
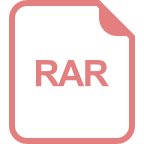
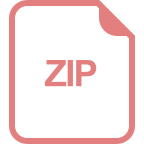
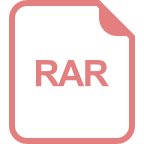
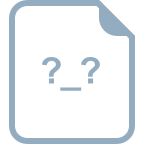