C语音编程实现将位于桌面的图片由bmp格式转为jpg格式,并对程序进行解释
时间: 2024-03-22 15:36:51 浏览: 14
好的,针对您的问题,我可以为您提供以下的C语言代码实现将位于桌面的图片由bmp格式转为jpg格式:
```c
#include <stdio.h>
#include <stdlib.h>
#include <jpeglib.h>
int main(int argc, char *argv[])
{
char *bmp_path = "C:\\Users\\username\\Desktop\\image.bmp"; // bmp图片的路径
char *jpg_path = "C:\\Users\\username\\Desktop\\image.jpg"; // 转换后的jpg图片路径
FILE *infile = fopen(bmp_path, "rb");
if (!infile)
{
printf("Error opening bmp file");
return 1;
}
fseek(infile, 0, SEEK_END);
size_t bmp_size = ftell(infile);
fseek(infile, 0, SEEK_SET);
unsigned char *bmp_buffer = (unsigned char*)malloc(bmp_size);
fread(bmp_buffer, bmp_size, 1, infile);
fclose(infile);
int width = *(int*)&bmp_buffer[18];
int height = *(int*)&bmp_buffer[22];
int depth = *(int*)&bmp_buffer[28];
if (depth != 24)
{
printf("Error: bmp image depth is not 24-bit");
free(bmp_buffer);
return 1;
}
struct jpeg_compress_struct cinfo;
struct jpeg_error_mgr jerr;
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_compress(&cinfo);
FILE *outfile = fopen(jpg_path, "wb");
if (!outfile)
{
printf("Error opening jpg file");
jpeg_destroy_compress(&cinfo);
free(bmp_buffer);
return 1;
}
jpeg_stdio_dest(&cinfo, outfile);
cinfo.image_width = width;
cinfo.image_height = height;
cinfo.input_components = 3;
cinfo.in_color_space = JCS_RGB;
jpeg_set_defaults(&cinfo);
jpeg_start_compress(&cinfo, TRUE);
JSAMPROW row_pointer[1];
row_pointer[0] = (JSAMPROW)calloc(width * 3, sizeof(JSAMPLE));
while (cinfo.next_scanline < cinfo.image_height)
{
int bmp_row = cinfo.image_height - cinfo.next_scanline - 1;
for (int i = 0; i < width; i++)
{
int bmp_index = bmp_row * (width * 3) + i * 3;
int jpg_index = i * 3;
row_pointer[0][jpg_index] = bmp_buffer[bmp_index + 2];
row_pointer[0][jpg_index + 1] = bmp_buffer[bmp_index + 1];
row_pointer[0][jpg_index + 2] = bmp_buffer[bmp_index];
}
jpeg_write_scanlines(&cinfo, row_pointer, 1);
}
jpeg_finish_compress(&cinfo);
fclose(outfile);
jpeg_destroy_compress(&cinfo);
free(bmp_buffer);
free(row_pointer[0]);
return 0;
}
```
对于这段代码,我可以进行以下的解释:
1. 包含必要的头文件:`stdio.h`、`stdlib.h`、`jpeglib.h`。
2. 定义了`main`函数,并传入了程序启动参数`argc`和`argv`。
3. 定义了bmp图片路径和转换后jpg图片路径。
4. 打开bmp文件并读取其内容到内存中。
5. 从bmp文件中读取图片的宽度、高度、位深等信息,并进行检查。
6. 创建jpeg文件并设置输出参数。
7. 逐行读取bmp文件并将其转换为jpg格式写入输出文件。
8. 关闭文件和释放内存。
需要注意的是,此代码使用的是jpeglib库进行图像的转换,因此需要先安装该库才能正常编译和运行。同时,该代码中的bmp图片的大小必须小于可用内存的大小,否则可能会出现内存溢出等错误。
相关推荐
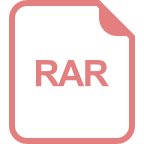
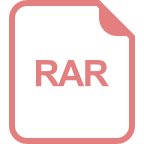
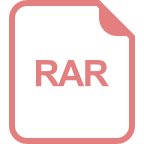














