用c++语言书写一个排序函数,输入任意类型的一组数,将其作为摸排表函数的参数输入,调用书写的排序模板函数,对数据排序,然后输出。
时间: 2024-05-02 20:22:50 浏览: 67
好的,请稍等一下,我会为您提供一个基于C语言的排序函数。
以下是基于C语言的排序函数:
```c
#include <stdio.h>
// 插入排序函数
void insertionSort(void *locations, int numOfElements, int elementSize, int (*compare)(const void *, const void *)) {
int i, j;
void *temp = (void *)malloc(elementSize);
for (i = 1; i < numOfElements; i++) {
memcpy(temp, locations + i * elementSize, elementSize);
j = i - 1;
while (j >= 0 && compare(locations + j * elementSize, temp) > 0) {
memcpy(locations + (j + 1) * elementSize, locations + j * elementSize, elementSize);
j--;
}
memcpy(locations + (j + 1) * elementSize, temp, elementSize);
}
free(temp);
}
// 比较函数
int compare(const void *a, const void *b) {
int *pa = (int *)a;
int *pb = (int *)b;
return *pa - *pb;
}
int main() {
int i;
int data[] = { 3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5 };
int numOfElements = sizeof(data) / sizeof(data[0]);
insertionSort((void *)data, numOfElements, sizeof(int), compare);
for (i = 0; i < numOfElements; i++) {
printf("%d ", data[i]);
}
printf("\n");
return 0;
}
```
以上是一个基于插入排序的函数,可以输入任何类型的一组数,输出升序排列的结果。其中,`insertionSort()`函数可以对不同类型的元素进行排序,而比较函数`compare()`则是对整型数据进行比较的示例。
注意:本程序只是一个示例,如果您需要对其他类型的数据进行排序,需要对相应的数据类型进行相应的修改。
阅读全文
相关推荐
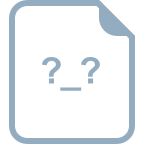
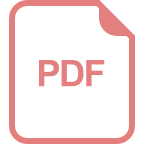
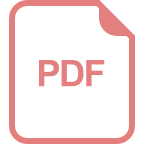
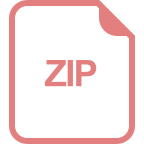
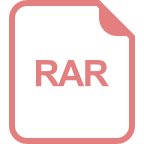
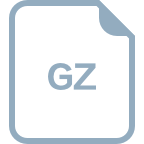
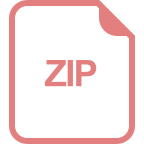
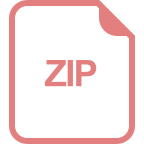
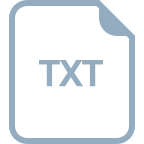
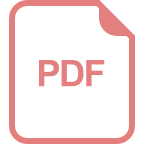
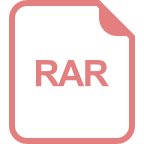
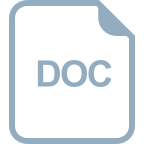
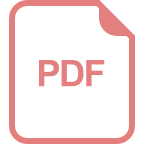
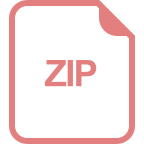
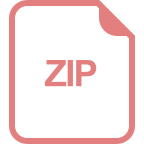
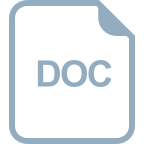