创建一个 Library类,包含一个 Book向量和一个Patron向量。定义一个名为Transaction 的 struet,包含一个Book对象、一个Patron对象和一个本章中定义的Date对象,表示借阅记录。在 Library 类中定义一个Transaction向量。定义向图书馆添加图书、添加读者以及借出书籍的函数。当一个读者借出一本书时,保证Library对象中有此读者和这本书的记录,否则报告错误。然后检查读者是否欠费,如果欠费就报告一个错误,否则创建一个Transaction对象,将其放人Transaction向量中。定义一个返回包含所有欠费读者姓名的向量的函数。该软件是多文件程序,按文件给出代码,在主测试文件中设置菜单,由用户手动录入图书信息,用C++设计实现,设计Book类,Patron类,Date类
时间: 2024-02-13 10:06:04 浏览: 218
以下是多文件程序的代码实现:
首先是Date类的头文件 Date.h:
```c++
#ifndef DATE_H
#define DATE_H
class Date {
private:
int year, month, day;
public:
Date(int y, int m, int d);
int getYear() const;
int getMonth() const;
int getDay() const;
};
#endif
```
Date类的实现文件 Date.cpp:
```c++
#include "Date.h"
Date::Date(int y, int m, int d) {
year = y;
month = m;
day = d;
}
int Date::getYear() const {
return year;
}
int Date::getMonth() const {
return month;
}
int Date::getDay() const {
return day;
}
```
接下来是Book类的头文件 Book.h:
```c++
#ifndef BOOK_H
#define BOOK_H
#include <string>
class Book {
private:
std::string title;
std::string author;
std::string isbn;
bool is_available;
public:
Book(std::string t, std::string a, std::string i);
std::string getTitle() const;
std::string getAuthor() const;
std::string getIsbn() const;
bool getAvailability() const;
void setAvailability(bool b);
};
#endif
```
Book类的实现文件 Book.cpp:
```c++
#include "Book.h"
Book::Book(std::string t, std::string a, std::string i) {
title = t;
author = a;
isbn = i;
is_available = true;
}
std::string Book::getTitle() const {
return title;
}
std::string Book::getAuthor() const {
return author;
}
std::string Book::getIsbn() const {
return isbn;
}
bool Book::getAvailability() const {
return is_available;
}
void Book::setAvailability(bool b) {
is_available = b;
}
```
接下来是Patron类的头文件 Patron.h:
```c++
#ifndef PATRON_H
#define PATRON_H
#include <string>
class Patron {
private:
std::string name;
double fees;
public:
Patron(std::string n);
std::string getName() const;
double getFees() const;
void addFee(double f);
};
#endif
```
Patron类的实现文件 Patron.cpp:
```c++
#include "Patron.h"
Patron::Patron(std::string n) {
name = n;
fees = 0.0;
}
std::string Patron::getName() const {
return name;
}
double Patron::getFees() const {
return fees;
}
void Patron::addFee(double f) {
fees += f;
}
```
最后是Library类的头文件 Library.h:
```c++
#ifndef LIBRARY_H
#define LIBRARY_H
#include <vector>
#include "Book.h"
#include "Patron.h"
#include "Date.h"
struct Transaction {
Book book;
Patron patron;
Date date;
};
class Library {
private:
std::vector<Book> books;
std::vector<Patron> patrons;
std::vector<Transaction> transactions;
public:
void addBook(Book b);
void addPatron(Patron p);
void lendBook(Book b, Patron p, Date d);
std::vector<std::string> getDebtors() const;
};
#endif
```
Library类的实现文件 Library.cpp:
```c++
#include "Library.h"
void Library::addBook(Book b) {
books.push_back(b);
}
void Library::addPatron(Patron p) {
patrons.push_back(p);
}
void Library::lendBook(Book b, Patron p, Date d) {
bool book_found = false;
bool patron_found = false;
int book_index = 0;
int patron_index = 0;
// Check if book exists
for (int i = 0; i < books.size(); i++) {
if (books[i].getIsbn() == b.getIsbn()) {
book_found = true;
book_index = i;
break;
}
}
// Check if patron exists
for (int i = 0; i < patrons.size(); i++) {
if (patrons[i].getName() == p.getName()) {
patron_found = true;
patron_index = i;
break;
}
}
// If book or patron not found, report error
if (!book_found || !patron_found) {
std::cout << "Error: book or patron not found" << std::endl;
return;
}
// Check if book is available
if (!books[book_index].getAvailability()) {
std::cout << "Error: book is not available" << std::endl;
return;
}
// Check if patron owes fees
if (patrons[patron_index].getFees() > 0.0) {
std::cout << "Error: patron owes fees" << std::endl;
return;
}
// Lend book and record transaction
books[book_index].setAvailability(false);
Transaction t = { b, p, d };
transactions.push_back(t);
}
std::vector<std::string> Library::getDebtors() const {
std::vector<std::string> debtors;
for (int i = 0; i < patrons.size(); i++) {
if (patrons[i].getFees() > 0.0) {
debtors.push_back(patrons[i].getName());
}
}
return debtors;
}
```
最后是主测试文件 main.cpp,包含一个简单的菜单:
```c++
#include <iostream>
#include "Library.h"
int main() {
Library library;
while (true) {
std::cout << "1. Add book" << std::endl;
std::cout << "2. Add patron" << std::endl;
std::cout << "3. Lend book" << std::endl;
std::cout << "4. List debtors" << std::endl;
std::cout << "5. Exit" << std::endl;
std::cout << "Enter choice: ";
int choice;
std::cin >> choice;
if (choice == 1) {
std::cout << "Enter title: ";
std::string title;
std::cin >> title;
std::cout << "Enter author: ";
std::string author;
std::cin >> author;
std::cout << "Enter ISBN: ";
std::string isbn;
std::cin >> isbn;
Book book(title, author, isbn);
library.addBook(book);
std::cout << "Book added" << std::endl;
}
else if (choice == 2) {
std::cout << "Enter name: ";
std::string name;
std::cin >> name;
Patron patron(name);
library.addPatron(patron);
std::cout << "Patron added" << std::endl;
}
else if (choice == 3) {
std::cout << "Enter ISBN: ";
std::string isbn;
std::cin >> isbn;
Book book("", "", isbn);
std::cout << "Enter patron name: ";
std::string name;
std::cin >> name;
Patron patron(name);
std::cout << "Enter date (year month day): ";
int year, month, day;
std::cin >> year >> month >> day;
Date date(year, month, day);
library.lendBook(book, patron, date);
}
else if (choice == 4) {
std::vector<std::string> debtors = library.getDebtors();
if (debtors.size() == 0) {
std::cout << "No debtors" << std::endl;
}
else {
std::cout << "Debtors:" << std::endl;
for (int i = 0; i < debtors.size(); i++) {
std::cout << debtors[i] << std::endl;
}
}
}
else if (choice == 5) {
break;
}
}
return 0;
}
```
阅读全文
相关推荐
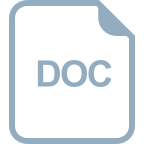
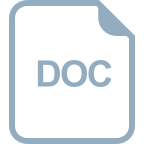
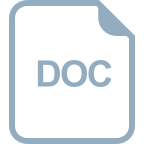

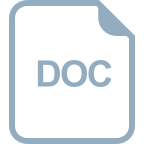
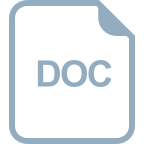
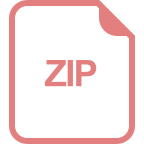
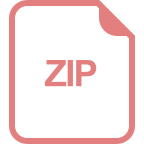
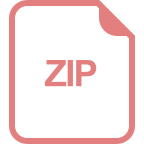
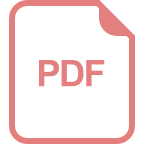
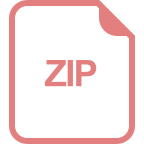
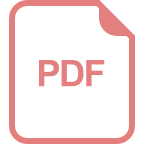
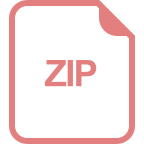
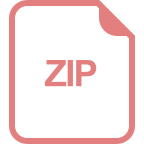
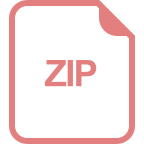
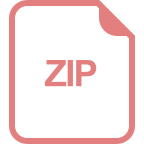
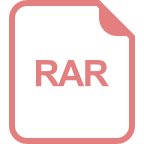