将一个顺序表里的所有元素以反转的方式加入到一个链表中
时间: 2023-12-20 19:52:16 浏览: 26
假设顺序表中的元素类型为 int,链表节点的结构体定义如下:
```c++
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
```
则可以先将顺序表中的所有元素逆序存放到一个新的顺序表中,然后遍历新的顺序表,依次将每个元素创建成链表节点,并将其插入到链表的头部,即可得到反转后的链表。具体代码实现如下:
```c++
#include <iostream>
#include <vector>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
ListNode* reverseList(vector<int>& nums) {
int n = nums.size();
vector<int> reverse_nums(n);
for (int i = 0; i < n; ++i) {
reverse_nums[n - i - 1] = nums[i];
}
ListNode* head = nullptr;
for (int i = 0; i < n; ++i) {
ListNode* node = new ListNode(reverse_nums[i]);
node->next = head;
head = node;
}
return head;
}
void printList(ListNode* head) {
while (head != nullptr) {
cout << head->val << " ";
head = head->next;
}
cout << endl;
}
int main() {
vector<int> nums = {1, 2, 3, 4, 5};
ListNode* head = reverseList(nums);
printList(head); // 输出:5 4 3 2 1
return 0;
}
```
相关推荐
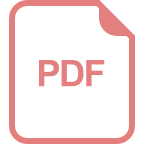














