请帮我注释下面代码def main(): global gaming, remain_ball, stage,score while True: if gaming: if stage==3: jk=score_font.render("闯关成功",True,PURPLE) screen.blit(jk,[310,250]) for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() if event.type == pygame.MOUSEBUTTONDOWN and event.button == 1: if remain_ball > 0: new_ball = Ball(0) remain_ball -= 1 score+=1 if check_collide(new_ball): gaming = False else: restart() game_stage() update() pygame.display.update() fclock.tick(fps)
时间: 2024-02-10 22:34:41 浏览: 74
这段代码主要实现了一个游戏的主循环逻辑
- `main()` 函数定义了游戏的主循环逻辑
- `while True:` 表示游戏会一直执行下去,直到被手动关闭
- `if gaming:` 判断当前是否处于游戏状态
- `if stage==3:` 判断当前游戏是否已经通关
- `for event in pygame.event.get():` 获取当前的事件队列
- `if event.type == pygame.QUIT:` 判断当前是否接收到了关闭窗口的事件
- `if event.type == pygame.MOUSEBUTTONDOWN and event.button == 1:` 判断当前是否接收到了鼠标左键点击的事件
- `if remain_ball > 0:` 判断当前是否还有剩余的球可以发射
- `new_ball = Ball(0)` 创建一个新的球对象
- `remain_ball -= 1` 减少剩余球数
- `score+=1` 增加得分
- `if check_collide(new_ball):` 检测当前球是否与其他物体发生碰撞
- `gaming = False` 如果发生碰撞,则游戏结束
- `else:` 如果当前不处于游戏状态,则执行 `restart()` 函数,重新开始游戏
- `game_stage()` 更新当前游戏的阶段状态
- `update()` 更新游戏画面
- `pygame.display.update()` 更新屏幕显示
- `fclock.tick(fps)` 控制游戏帧率,以保证游戏运行流畅
相关问题
帮我注释下面代码class Ball: """ 针头 """ def __init__(self, angle): self.x = x0 self.y = y0 + length self.center = (self.x, self.y) self.radius = 12 self.angle = angle ball_group.append(self) def draw(self, surface): pygame.draw.line(surface, WHITE, shaft, self.center, 2) pygame.draw.circle(surface, WHITE, self.center, 12) def move(self, speed): """ 围绕转轴做圆周运动 :param speed: 转动的角速度 :return: """ if self.angle < 2 * math.pi: self.angle += speed else: self.angle = self.angle - 2 * math.pi self.x = x0 - length * math.sin(self.angle) self.y = y0 + length * math.cos(self.angle) self.center = (self.x, self.y) def check_collide(new_ball): for ball in ball_group: distance = math.sqrt(abs(ball.x - new_ball.x) ** 2 + abs(ball.y - new_ball.y) ** 2) if ball is not new_ball and distance <= new_ball.radius * 2: return True return False def game_init(): global stage, gaming, remain_ball, stage_pass,score if stage == len(all_stage): stage = 1 elif stage_pass: stage += 1 ball_group.clear() for a in all_stage[stage - 1]: b = Ball(a) remain_ball = ball_num[stage - 1] score=0 pygame.time.delay(200) gaming = True stage_pass = False button = Button('重新开始', color=(220, 0, 0)) button.rect.center = shaft button.click_connect(game_init) def restart(): for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() button.get_click(event) def game_stage(): global remain_ball, stage, gaming, stage_pass,score if remain_ball == 0 and gaming: stage_pass = True gaming = False
这段代码是一个 Python 类的定义,名为 `Ball`,表示一个球的属性和行为。下面是对每个方法的注释:
- `__init__`: 初始化方法,传入一个 `angle` 参数表示球相对于垂直线的旋转角度,设置球的初始坐标、圆心、半径和角度,并将球添加到球组中。
- `draw`: 绘制球的方法,传入一个 `surface` 参数表示绘制球的表面,使用 Pygame 库的 `draw` 函数绘制球的轴和圆。
- `move`: 移动球的方法,传入一个 `speed` 参数表示球的角速度,根据角度计算球的新坐标和圆心,实现球的圆周运动。
- `check_collide`: 检查球碰撞的方法,传入一个 `new_ball` 参数表示一个新的球,遍历球组中的每个球,计算新球与其他球之间的距离,判断是否发生碰撞。
- `game_init`: 初始化游戏的方法,设置当前游戏的关卡、剩余球数、得分等变量,清空球组,根据当前关卡生成新的球,并将游戏状态设置为进行中。
- `restart`: 重新开始游戏的方法,处理 Pygame 库的事件,包括退出游戏和点击重新开始按钮。
- `game_stage`: 游戏关卡的方法,根据剩余球数和游戏状态判断游戏是否结束或通关,更新游戏状态和得分等变量。
wangyize@wangyize-Victus-BY-HP-16-1-Inch-Gaming-Laptop-PC:~/catkin_ws$ roslaunch smartcar smartcar_display.rviz.launch ... logging to /home/wangyize/.ros/log/8f67e756-fa27-11ed-8cf9-09137e54c687/roslaunch-wangyize-Victus-BY-HP-16-1-Inch-Gaming-Laptop-PC-39585.log Checking log directory for disk usage. This may take a while. Press Ctrl-C to interrupt Done checking log file disk usage. Usage is <1GB. RLException: Invalid roslaunch XML syntax: not well-formed (invalid token): line 2, column 1 The traceback for the exception was written to the log file
根据你提供的信息,roslaunch启动失败了,并报告了一个错误。错误信息显示了不正确的XML语法,可能是由于在第2行第1列出现了一个无效的标记导致的。
你可以尝试检查smartcar_display.rviz.launch文件中的XML语法并确保其正确性。你还可以尝试在终端中使用roslaunch命令启动ROS节点,以查看是否会出现类似的错误信息,并进一步排除问题。
阅读全文
相关推荐
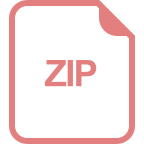
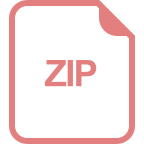
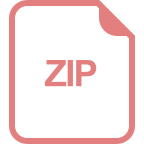
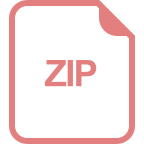
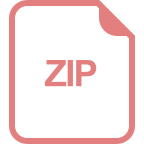
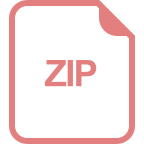
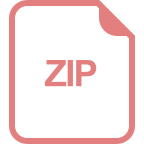
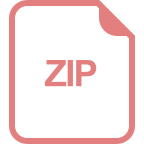
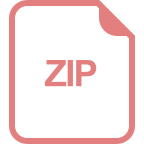
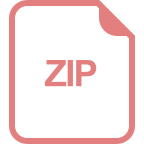
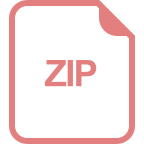
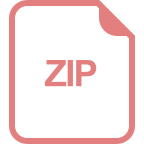
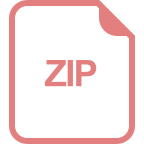
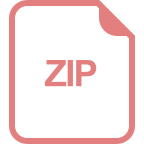
