C++函数指针和std::is_same用来在数据格式fp_t为float时用cublasSaxpy,为double时用cublasDaxpy
时间: 2023-06-13 13:08:49 浏览: 52
可以使用函数指针和std::is_same来实现在数据类型为float时使用cublasSaxpy,为double时使用cublasDaxpy的功能。具体实现过程如下:
1. 首先定义一个模板函数,用来根据模板参数T来选择对应的函数指针类型和函数名:
```c++
template<typename T>
struct FuncPtr {
using type = void (*)(int, T, T*, int, T*, int);
static constexpr const char* name = std::is_same_v<T, float> ? "cublasSaxpy" : "cublasDaxpy";
};
```
这里使用了std::is_same来判断T是否为float类型,如果是则函数指针类型为void (*)(int, float, float*, int, float*, int),函数名为"cublasSaxpy";否则函数指针类型为void (*)(int, double, double*, int, double*, int),函数名为"cublasDaxpy"。
2. 然后在使用函数指针的地方,使用FuncPtr::type来定义函数指针类型,使用FuncPtr::name来获取函数名:
```c++
using fp_t = float;
FuncPtr<fp_t>::type func = reinterpret_cast<FuncPtr<fp_t>::type>(dlsym(handle, FuncPtr<fp_t>::name));
```
这里使用了dlsym来获取函数指针,具体的获取方法可以参考相关文档。
3. 最后就可以使用函数指针来调用对应的函数了:
```c++
func(n, alpha, x, incx, y, incy);
```
完整的代码示例如下:
```c++
#include <iostream>
#include <dlfcn.h>
#include <type_traits>
template<typename T>
struct FuncPtr {
using type = void (*)(int, T, T*, int, T*, int);
static constexpr const char* name = std::is_same_v<T, float> ? "cublasSaxpy" : "cublasDaxpy";
};
int main() {
void* handle = dlopen("libcublas.so", RTLD_LAZY);
if (!handle) {
std::cerr << "Failed to load libcublas.so: " << dlerror() << std::endl;
return -1;
}
using fp_t = float;
FuncPtr<fp_t>::type func = reinterpret_cast<FuncPtr<fp_t>::type>(dlsym(handle, FuncPtr<fp_t>::name));
if (!func) {
std::cerr << "Failed to load function " << FuncPtr<fp_t>::name << ": " << dlerror() << std::endl;
return -1;
}
const int n = 10;
const fp_t alpha = 1.0;
fp_t x[n] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
fp_t y[n] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
const int incx = 1, incy = 1;
func(n, alpha, x, incx, y, incy);
for (int i = 0; i < n; ++i) {
std::cout << y[i] << " ";
}
std::cout << std::endl;
dlclose(handle);
return 0;
}
```
相关推荐
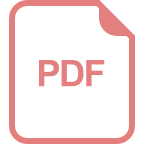
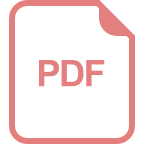
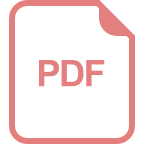














