写一个程序,创建一个管道,然后在父子进程间“ping-pong”一个字节,即父进程从键盘读取一个字符,通过管道写给子进程,子进程收到后再发回给父进程,父进程将这个字符显示在屏幕上。
时间: 2023-06-08 11:04:09 浏览: 131
好的,这是一个编程问题,我可以为您提供帮助。以下是C语言的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
int main(void)
{
int pipefd[2];
pid_t cpid;
char buf;
if (pipe(pipefd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
cpid = fork();
if (cpid == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
if (cpid == 0) { /* 子进程 */
close(pipefd[1]); /* 关闭写端 */
while (read(pipefd[0], &buf, 1) > 0)
write(STDOUT_FILENO, &buf, 1);
write(STDOUT_FILENO, "\n", 1);
close(pipefd[0]);
_exit(EXIT_SUCCESS);
} else { /* 父进程 */
close(pipefd[0]); /* 关闭读端 */
while (read(STDIN_FILENO, &buf, 1) > 0)
write(pipefd[1], &buf, 1);
close(pipefd[1]); /* 关闭写端,向子进程发送 EOF */
wait(NULL); /* 等待子进程退出 */
exit(EXIT_SUCCESS);
}
}
```
该程序使用了系统调用pipe、fork、read、write、close和wait。在父子进程间创建了一个匿名管道,并进行往来传输一个字节。父进程从键盘读取一个字符,通过管道写给子进程。子进程读取后再发回给父进程,父进程将这个字符显示在屏幕上。
您可以参考上述代码来解决您的问题。希望能对您有所帮助!
相关推荐
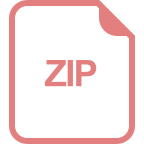
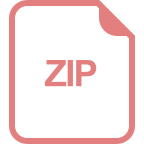














