@不是要和DP或SP配合使用吗
时间: 2023-08-09 18:09:54 浏览: 40
是的,ChitGPT是一个类ChatGPT的AI,可以与您进行对话。但是,为了更好地使用ChitGPT,建议结合DP(Dialogue Policy)或SP(Slot Filling)等对话管理技术一起使用,以便更好地控制对话流程和提高对话效果。这些技术可以帮助您更好地管理和处理对话中的语言和交互信息。
相关问题
android dp sp px
在 Android 设备上,像素(Pixel)是指显示屏上的最小颜色点,而 dp、sp、px 则用于描述在不同分辨率的设备上,一个视觉元素的尺寸和位置。
- dp(density-independent pixels):密度无关像素。是一种基于屏幕密度的抽象单位,它可以让布局在不同的设备上有相同的显示效果。1dp 对应的像素数取决于设备的屏幕密度。通常,在设计 UI 时应使用 dp 单位。
- sp(scale-independent pixels):与 dp 类似,但用于描述文字大小。与 dp 不同的是,用户可以在系统设置中调整字体大小,因此 sp 的值会随着用户在系统设置中的调整而发生变化。
- px(pixels):像素。是屏幕上的最小显示单位,1px 对应一个实际的像素点。在设计 UI 时,应该尽量避免使用 px,因为在不同分辨率的设备上会导致 UI 显示效果不一致。
在布局文件中,可以使用 dp 和 sp 单位来设置视觉元素的尺寸和位置,例如:
```
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="16sp"
android:padding="8dp"
android:text="Hello, world!" />
```
在代码中,可以使用以下方法将 dp 或 sp 转换为 px:
```
// 将 dp 转换为 px
int px = (int) TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_DIP, 16, getResources().getDisplayMetrics());
// 将 sp 转换为 px
int px = (int) TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_SP, 16, getResources().getDisplayMetrics());
```
GTX1650跑深度学习只能使用DP模式吗
不一定,GTX 1650显卡支持DP(DisplayPort)模式和HDMI模式,而且在深度学习中一般都是使用CUDA进行计算,而不是使用显卡的视频输出。因此,使用DP模式或HDMI模式对于深度学习的计算并没有太大的影响。
DP模式和HDMI模式的主要区别在于带宽和支持的最大分辨率等方面,DP模式的带宽比较大,支持的分辨率也比较高,因此在连接高分辨率显示器或多个显示器时,DP模式可能更为适合。但是在深度学习中,显卡的计算能力和显存大小等更为重要,因此DP模式和HDMI模式并没有太大的差别。
需要注意的是,如果使用DP模式或HDMI模式连接显示器时,需要选择正确的线缆和适配器,以确保信号传输的稳定性和可靠性。同时,在进行深度学习计算时,也需要根据具体的算法和模型选择正确的GPU加速库和计算框架,以充分发挥显卡的计算能力。
相关推荐
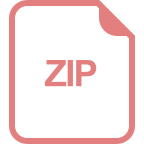
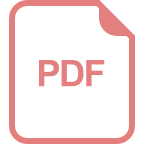
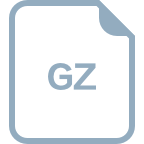












