Android忘记密码功能实现
时间: 2023-08-05 18:50:56 浏览: 129
实现 Android 忘记密码功能,可以按照以下步骤进行:
1. 在登录页面添加一个“忘记密码”按钮,当用户点击按钮时,跳转到密码重置页面。
2. 在密码重置页面中,让用户输入其注册时使用的邮箱地址,并在页面上添加一个“发送重置链接”的按钮。
3. 当用户点击发送按钮时,应该向输入的邮箱地址发送包含重置密码链接的电子邮件。
4. 用户收到邮件后,可以点击链接跳转到密码重置页面,并重新设置密码。
5. 在密码重置页面中,应该包含一个密码输入框和一个确认密码输入框。用户输入新密码并确认后,点击“确认”按钮即可完成密码重置。
6. 对于一些安全性较高的应用,还可以添加短信验证或者安全问题验证等额外的验证方式。
以上是实现 Android 忘记密码功能的基本步骤,具体实现过程可以根据实际需求进行调整。
相关问题
Android忘记密码实现的具体代码
以下是一个简单的示例代码,展示了如何在 Android 应用程序中实现忘记密码功能:
1. 在布局文件中添加一个“忘记密码”按钮。
activity_login.xml:
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<EditText
android:id="@+id/edit_text_username"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="用户名"/>
<EditText
android:id="@+id/edit_text_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="密码"/>
<Button
android:id="@+id/button_login"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="登录"/>
<Button
android:id="@+id/button_forgot_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="忘记密码"/>
</LinearLayout>
```
2. 在 LoginActivity 中处理“忘记密码”按钮的点击事件,跳转到密码重置页面。
LoginActivity.java:
```java
public class LoginActivity extends AppCompatActivity {
private EditText mEditTextUsername;
private EditText mEditTextPassword;
private Button mButtonLogin;
private Button mButtonForgotPassword;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
mEditTextUsername = findViewById(R.id.edit_text_username);
mEditTextPassword = findViewById(R.id.edit_text_password);
mButtonLogin = findViewById(R.id.button_login);
mButtonForgotPassword = findViewById(R.id.button_forgot_password);
mButtonLogin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// 处理登录逻辑
}
});
mButtonForgotPassword.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
startActivity(new Intent(LoginActivity.this, ResetPasswordActivity.class));
}
});
}
}
```
3. 在 ResetPasswordActivity 中让用户输入其注册时使用的邮箱地址,并添加一个“发送重置链接”的按钮。
activity_reset_password.xml:
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<EditText
android:id="@+id/edit_text_email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="邮箱地址"/>
<Button
android:id="@+id/button_send_reset_link"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="发送重置链接"/>
</LinearLayout>
```
ResetPasswordActivity.java:
```java
public class ResetPasswordActivity extends AppCompatActivity {
private EditText mEditTextEmail;
private Button mButtonSendResetLink;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_reset_password);
mEditTextEmail = findViewById(R.id.edit_text_email);
mButtonSendResetLink = findViewById(R.id.button_send_reset_link);
mButtonSendResetLink.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// 发送重置邮件的逻辑
}
});
}
}
```
4. 在发送重置邮件的逻辑中,使用 JavaMail API 发送包含重置密码链接的电子邮件。
```java
public void sendResetEmail(String emailAddress) throws MessagingException {
Properties props = new Properties();
props.put("mail.smtp.host", "smtp.gmail.com");
props.put("mail.smtp.port", "587");
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
Session session = Session.getInstance(props, new Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("your_email_address", "your_email_password");
}
});
String resetLink = "https://www.example.com/reset_password?email=" + emailAddress;
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("your_email_address"));
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(emailAddress));
message.setSubject("密码重置链接");
message.setText("请点击以下链接重置您的密码:" + resetLink);
Transport.send(message);
}
```
在实际应用中,需要根据具体需求进行调整和完善。
在Android Studio实现找回密码
在Android Studio中实现找回密码功能可以通过以下步骤进行:
1. 创建找回密码界面布局文件:在res/layout目录下创建一个新的XML文件,例如"activity_forgot_password.xml",并在该文件中设计找回密码界面的布局,包括输入框和按钮等组件。
2. 创建找回密码的Activity类:在Java包中创建一个新的Java类,例如"ForgotPasswordActivity.java",并在该类中编写找回密码功能的逻辑代码。
3. 在找回密码Activity中获取用户输入的邮箱或手机号:在找回密码Activity的代码中,通过findViewById方法获取用户输入的邮箱或手机号的EditText组件,并获取用户输入的值。
4. 实现找回密码的逻辑:根据用户输入的邮箱或手机号,可以通过发送重置密码的请求到服务器,或者通过本地数据库查询用户信息来实现找回密码的逻辑。具体的实现方式取决于你的后端服务或数据库设计。
5. 处理找回密码的结果:根据服务器返回的结果或数据库查询的结果,可以在找回密码Activity中显示相应的提示信息,例如密码重置成功或失败的提示。
6. 在登录界面中添加找回密码的入口:在登录界面的布局文件中,添加一个"忘记密码"的TextView或Button,并在点击事件中跳转到找回密码的Activity。
以下是一个示例代码,演示了如何在Android Studio中实现找回密码功能:
```java
// ForgotPasswordActivity.java
public class ForgotPasswordActivity extends AppCompatActivity {
private EditText emailEditText;
private Button resetButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_forgot_password);
emailEditText = findViewById(R.id.emailEditText);
resetButton = findViewById(R.id.resetButton);
resetButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String email = emailEditText.getText().toString();
// 发送重置密码的请求到服务器或查询本地数据库
// 处理找回密码的结果并显示相应的提示信息
}
});
}
}
```
```xml
<!-- activity_forgot_password.xml -->
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/emailEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Email or Phone" />
<Button
android:id="@+id/resetButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Reset Password" />
</LinearLayout>
```
相关推荐
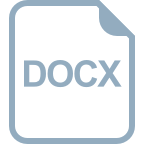
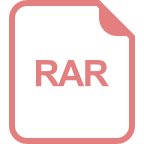












