统计在一个输入字符串中各个不同字符出现的次数,按最多到最少的顺序依次打印c++
时间: 2024-09-14 20:07:58 浏览: 73
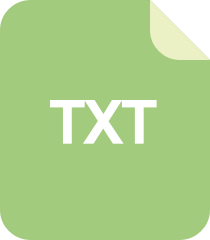
统计文件中某字符出现的次数(c++)
在C++中统计输入字符串中各个不同字符出现的次数,并按出现次数最多到最少的顺序打印出来,可以通过使用`std::map`或`std::unordered_map`来实现。以下是实现该功能的一种方法:
1. 使用`std::unordered_map`来存储每个字符及其出现的次数。`unordered_map`在C++中是基于哈希表实现的,能够提供平均常数时间复杂度的查找效率。
2. 遍历输入的字符串,对于每个字符,更新`unordered_map`中对应字符的计数。
3. 将`unordered_map`中的元素复制到一个`vector`中,以便排序。
4. 使用标准库中的`sort`函数对`vector`中的元素按出现次数进行排序,可以通过自定义比较函数实现。
5. 遍历排序后的`vector`,按照出现次数从多到少打印字符及其计数。
示例代码如下:
```cpp
#include <iostream>
#include <unordered_map>
#include <vector>
#include <algorithm>
#include <utility> // for std::pair
int main() {
std::string input;
std::unordered_map<char, int> charCount;
std::vector<std::pair<char, int>> countVector;
// 获取输入字符串
std::getline(std::cin, input);
// 统计字符出现次数
for (char ch : input) {
if (ch != ' ') { // 假设空格不计入统计
charCount[ch]++;
}
}
// 将unordered_map中的数据转移到vector中
for (const auto &pair : charCount) {
countVector.push_back(pair);
}
// 根据出现次数对vector进行排序
std::sort(countVector.begin(), countVector.end(),
[](const std::pair<char, int> &a, const std::pair<char, int> &b) {
return a.second > b.second; // 按出现次数降序排序
});
// 打印排序后的字符和出现次数
for (const auto &pair : countVector) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
在上述代码中,我们首先读取一个字符串,然后统计每个字符的出现次数,并存储在`unordered_map`中。之后,我们将这些元素转移到`vector`中,并按出现次数进行排序。最后,遍历`vector`并打印结果。
阅读全文
相关推荐
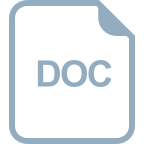
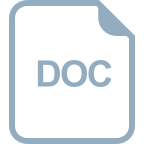
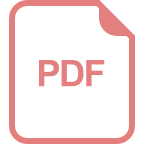
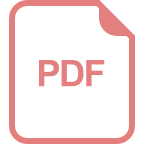
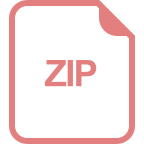
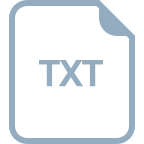
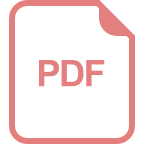
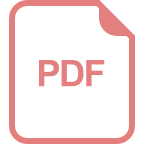
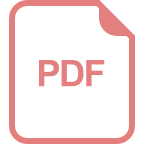
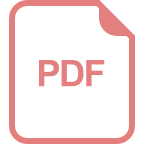
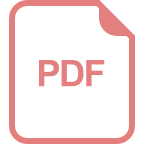
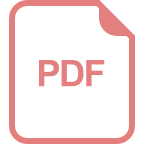
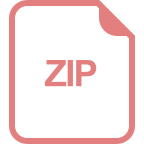
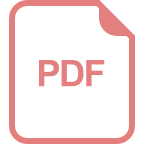
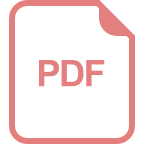