有3个学生,每个学生的数据包括学号,姓名,3门课的成绩。请设计以下3个函数,实现数据的输入,输出等操作。 (1)设计一个函数,输入n个学生的成绩。 (2)设计一个函数,输出n个学生的成绩。 (3)设计一个函数,找出n个学生中总分最高的学生。
时间: 2024-05-09 17:18:25 浏览: 196
好的,我明白了,我们开始第一个问题的解答:
首先,我们可以定义一个学生类,包括学号、姓名、三门课的成绩,如下所示:
```python
class Student:
def __init__(self, stu_id, name, score1, score2, score3):
self.stu_id = stu_id
self.name = name
self.score1 = score1
self.score2 = score2
self.score3 = score3
```
接下来,我们可以定义一个函数用于输入n个学生的成绩,代码如下:
```python
def input_scores(n):
students = []
for i in range(n):
stu_id = input("请输入第{}个学生的学号:".format(i+1))
name = input("请输入第{}个学生的姓名:".format(i+1))
score1 = float(input("请输入第{}个学生的第一门课成绩:".format(i+1)))
score2 = float(input("请输入第{}个学生的第二门课成绩:".format(i+1)))
score3 = float(input("请输入第{}个学生的第三门课成绩:".format(i+1)))
student = Student(stu_id, name, score1, score2, score3)
students.append(student)
return students
```
以上函数中,我们使用了一个for循环,依次输入每个学生的信息,并将其存储在一组列表中。
接着,我们定义一个函数用于输出n个学生的成绩,代码如下:
```python
def output_scores(students):
for student in students:
print("学号:{}\t姓名:{}\t成绩:\t{}\t{}\t{}".format(student.stu_id, student.name, student.score1, student.score2, student.score3))
```
该函数中,我们使用一个for循环遍历每个学生,并将其成绩逐一输出。
最后,我们定义一个函数用于找出n个学生中总分最高的学生,代码如下:
```python
def find_top_student(students):
top_student = students[0]
top_score = students[0].score1 + students[0].score2 + students[0].score3
for student in students:
total_score = student.score1 + student.score2 + student.score3
if total_score > top_score:
top_score = total_score
top_student = student
return top_student
```
该函数中,我们使用了一个for循环遍历每个学生,并找出总分最高的学生。
以上就是解决该问题的全部代码,你可以将其整合在一起,如下所示:
```python
class Student:
def __init__(self, stu_id, name, score1, score2, score3):
self.stu_id = stu_id
self.name = name
self.score1 = score1
self.score2 = score2
self.score3 = score3
def input_scores(n):
students = []
for i in range(n):
stu_id = input("请输入第{}个学生的学号:".format(i+1))
name = input("请输入第{}个学生的姓名:".format(i+1))
score1 = float(input("请输入第{}个学生的第一门课成绩:".format(i+1)))
score2 = float(input("请输入第{}个学生的第二门课成绩:".format(i+1)))
score3 = float(input("请输入第{}个学生的第三门课成绩:".format(i+1)))
student = Student(stu_id, name, score1, score2, score3)
students.append(student)
return students
def output_scores(students):
for student in students:
print("学号:{}\t姓名:{}\t成绩:\t{}\t{}\t{}".format(student.stu_id, student.name, student.score1, student.score2, student.score3))
def find_top_student(students):
top_student = students[0]
top_score = students[0].score1 + students[0].score2 + students[0].score3
for student in students:
total_score = student.score1 + student.score2 + student.score3
if total_score > top_score:
top_score = total_score
top_student = student
return top_student
n = int(input("请输入学生数:"))
students = input_scores(n)
output_scores(students)
top_student = find_top_student(students)
print("总分最高的学生:学号:{}\t姓名:{}\t成绩:\t{}\t{}\t{}".format(top_student.stu_id, top_student.name, top_student.score1, top_student.score2, top_student.score3))
```
阅读全文
相关推荐
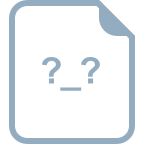
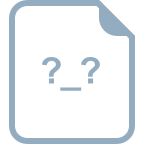
















