jQuery的方法实现轮播图,实现的轮播图最少由3幅图组成,每隔一段时间自动切换到下一幅图;鼠标停留时显示左右切换的按钮,点击左右按钮可以切换到上一幅或下一幅图;点击图下面的圆形下标,切换到对应的图。图片切换需无缝切换,首尾图片切换时无违和感的代码
时间: 2024-03-14 08:43:49 浏览: 104
好的,以下是基于jQuery实现轮播图的代码:
HTML结构:
```
<div class="carousel">
<ul class="carousel-list">
<li><img src="img1.jpg" alt=""></li>
<li><img src="img2.jpg" alt=""></li>
<li><img src="img3.jpg" alt=""></li>
</ul>
<ul class="carousel-nav">
<li class="active"></li>
<li></li>
<li></li>
</ul>
<a href="#" class="carousel-prev"></a>
<a href="#" class="carousel-next"></a>
</div>
```
CSS样式:
```
.carousel {
position: relative;
width: 600px;
height: 400px;
overflow: hidden;
}
.carousel-list {
position: absolute;
left: 0;
top: 0;
width: 1800px;
height: 400px;
margin: 0;
padding: 0;
list-style: none;
}
.carousel-list li {
float: left;
}
.carousel-nav {
position: absolute;
left: 50%;
bottom: 20px;
transform: translateX(-50%);
margin: 0;
padding: 0;
list-style: none;
}
.carousel-nav li {
display: inline-block;
width: 10px;
height: 10px;
margin: 0 5px;
border-radius: 50%;
background-color: #ccc;
cursor: pointer;
}
.carousel-nav li.active {
background-color: #f00;
}
.carousel-prev, .carousel-next {
position: absolute;
top: 50%;
transform: translateY(-50%);
width: 20px;
height: 20px;
background-color: #ccc;
opacity: 0.5;
cursor: pointer;
}
.carousel-prev {
left: 20px;
}
.carousel-next {
right: 20px;
}
```
jQuery代码:
```
$(function () {
var $carousel = $('.carousel'),
$list = $carousel.find('.carousel-list'),
$items = $list.find('li'),
$nav = $carousel.find('.carousel-nav'),
$navItems = $nav.find('li'),
$prev = $carousel.find('.carousel-prev'),
$next = $carousel.find('.carousel-next'),
itemWidth = $items.outerWidth(true),
itemCount = $items.length,
currentIndex = 0,
isAnimating = false,
timer = null,
interval = 3000;
// 初始化
$list.css('width', itemWidth * itemCount);
$navItems.eq(currentIndex).addClass('active');
// 自动轮播
function play() {
timer = setInterval(function () {
next();
}, interval);
}
// 停止轮播
function stop() {
clearInterval(timer);
}
// 切换到下一张图片
function next() {
if (isAnimating) {
return;
}
isAnimating = true;
currentIndex++;
if (currentIndex >= itemCount) {
currentIndex = 0;
}
$navItems.removeClass('active').eq(currentIndex).addClass('active');
$list.animate({
left: -itemWidth * currentIndex
}, function () {
isAnimating = false;
});
}
// 切换到上一张图片
function prev() {
if (isAnimating) {
return;
}
isAnimating = true;
currentIndex--;
if (currentIndex < 0) {
currentIndex = itemCount - 1;
}
$navItems.removeClass('active').eq(currentIndex).addClass('active');
$list.animate({
left: -itemWidth * currentIndex
}, function () {
isAnimating = false;
});
}
// 点击圆形下标切换图片
$navItems.on('click', function () {
var index = $(this).index();
if (index === currentIndex) {
return;
}
currentIndex = index;
$navItems.removeClass('active').eq(currentIndex).addClass('active');
$list.animate({
left: -itemWidth * currentIndex
});
});
// 鼠标移入停止轮播,显示左右按钮
$carousel.on('mouseenter', function () {
stop();
$prev.stop().animate({
left: 0,
opacity: 1
});
$next.stop().animate({
right: 0,
opacity: 1
});
});
// 鼠标移出开始轮播,隐藏左右按钮
$carousel.on('mouseleave', function () {
play();
$prev.stop().animate({
left: -20,
opacity: 0.5
});
$next.stop().animate({
right: -20,
opacity: 0.5
});
});
// 点击左右按钮切换图片
$prev.on('click', function () {
prev();
});
$next.on('click', function () {
next();
});
// 开始轮播
play();
});
```
这份代码实现了基本的轮播图功能,包括自动轮播、鼠标移入移出、左右切换按钮、圆形下标切换等,图片切换无缝,首尾图片切换时也没有违和感。
阅读全文
相关推荐
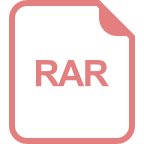
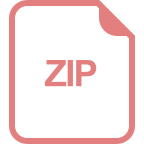
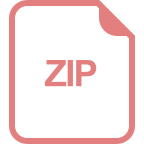
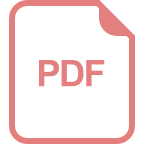
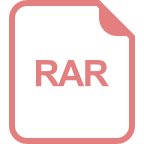
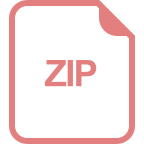
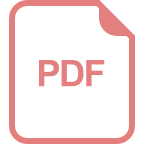
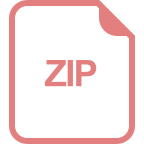
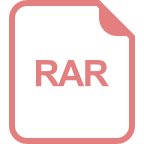
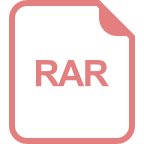
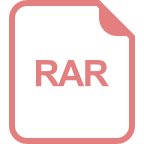
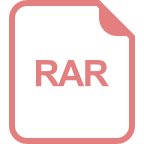
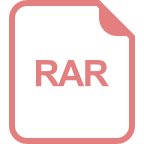