用c++实现维吉尼亚密码加解密
时间: 2023-09-24 19:11:17 浏览: 234
好的,维吉尼亚密码是一种多表密码,通过多个凯撒密码表的组合来提高加密强度。下面是用C++实现维吉尼亚密码加解密的示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
string encrypt(string plaintext, string key) {
string ciphertext = "";
int keyIndex = 0;
for (int i = 0; i < plaintext.length(); i++) {
char c = plaintext[i];
if (isalpha(c)) {
int keyChar = key[keyIndex] - 'a';
int shift = tolower(c) - 'a' + keyChar;
c = (shift % 26) + 'a';
keyIndex = (keyIndex + 1) % key.length();
}
ciphertext += c;
}
return ciphertext;
}
string decrypt(string ciphertext, string key) {
string plaintext = "";
int keyIndex = 0;
for (int i = 0; i < ciphertext.length(); i++) {
char c = ciphertext[i];
if (isalpha(c)) {
int keyChar = key[keyIndex] - 'a';
int shift = tolower(c) - 'a' - keyChar;
if (shift < 0) shift += 26;
c = shift + 'a';
keyIndex = (keyIndex + 1) % key.length();
}
plaintext += c;
}
return plaintext;
}
int main() {
string plaintext = "This is a secret message.";
string key = "secret";
string ciphertext = encrypt(plaintext, key);
cout << "Ciphertext: " << ciphertext << endl;
string decryptedText = decrypt(ciphertext, key);
cout << "Decrypted text: " << decryptedText << endl;
return 0;
}
```
在这个示例代码中,我们定义了两个函数`encrypt()`和`decrypt()`,分别用于加密和解密。这两个函数都接受两个字符串参数,分别是明文/密文和密钥。在加密和解密过程中,我们都需要遍历明文/密文中的每个字符,并根据密钥中的字符来计算移位量,从而实现加密和解密的过程。
阅读全文
相关推荐









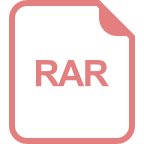

