n=collections.Counter(labels.values()).most_common()[-1][1]
时间: 2024-06-07 17:11:37 浏览: 8
这段代码的作用是统计一个字典(labels)中出现次数最多的元素出现的次数,并将结果赋值给变量n。
具体来说,这行代码使用了Python内置的collections模块中的Counter类,将字典labels转换为Counter对象,然后调用most_common()方法返回一个按照出现次数从大到小排序的元素列表。[-1]表示取出出现次数最多的元素,[1]表示取出该元素的出现次数。最终结果赋值给变量n。
相关问题
n = collections.Counter(labels.values()).most_common()[-1][1]
这行代码的作用是统计一个字典 `labels` 中出现次数最少的元素出现的次数。具体来说,`collections.Counter(labels.values())` 可以统计 `labels` 中每个元素出现的次数并返回一个字典,然后 `most_common()` 方法可以将字典中的元素按照出现次数从大到小排序并返回一个列表,最后 `[-1][1]` 取出了出现次数最少的元素出现的次数。
用python代码编写, 题目: 某银行有一份客户信用评估的数据集,包含了客户的一些特征信息以及他们是否违约的标签。现在要求你设计一个程序,使用决策树算法来建立一个信用评估模型,并对新客户进行信用评估。 要求: 设计一个程序,使用决策树算法(如ID3、C4.5或CART算法)建立一个信用评估模型。 提供必要的输入接口,例如特征数据和标签数据。 年龄 信用评级 是否有房产 25 高 是 40 低 否 35 中 否 20 高 是 30 中 否 45 低 否 50 高 是 28 中 是 32 低 否 38 中 是 对应是否违约: labels = [ "不违约", "违约", "不违约", "违约", "不违约", "不违约", "违约", "不违约", "不违约", "违约”] 现有新客户:37 中 否,用上述模型预测新客户是否违约。
好的,这是一个典型的分类问题,我们可以使用决策树算法来建立一个信用评估模型,然后对新客户进行预测。下面是一个使用ID3算法建立决策树的Python代码示例:
```python
from math import log2
from collections import Counter
class DecisionTree:
def __init__(self):
self.tree = None
def fit(self, X, y):
self.tree = self.build_tree(X, y)
def predict(self, X):
return [self.classify(self.tree, x) for x in X]
def build_tree(self, X, y):
if len(y) == 0:
return None
if len(set(y)) == 1:
return y[0]
if len(X[0]) == 1:
return Counter(y).most_common()[0][0]
best_feature, best_gain = self.choose_best_feature(X, y)
tree = {best_feature: {}}
for value in set(X[:, best_feature]):
sub_X, sub_y = self.split_dataset(X, y, best_feature, value)
tree[best_feature][value] = self.build_tree(sub_X, sub_y)
return tree
def choose_best_feature(self, X, y):
num_features = len(X[0])
base_entropy = self.entropy(y)
best_feature = -1
best_gain = 0
for i in range(num_features):
feature_list = X[:, i]
unique_values = set(feature_list)
new_entropy = 0
for value in unique_values:
sub_X, sub_y = self.split_dataset(X, y, i, value)
prob = len(sub_y) / len(y)
new_entropy += prob * self.entropy(sub_y)
info_gain = base_entropy - new_entropy
if info_gain > best_gain:
best_gain = info_gain
best_feature = i
return best_feature, best_gain
def split_dataset(self, X, y, feature, value):
sub_X = []
sub_y = []
for i in range(len(X)):
if X[i][feature] == value:
reduced_X = X[i][:feature]
reduced_X.extend(X[i][feature+1:])
sub_X.append(reduced_X)
sub_y.append(y[i])
return sub_X, sub_y
def entropy(self, labels):
num_labels = len(labels)
label_counts = Counter(labels)
probs = [label_counts[label] / num_labels for label in label_counts]
entropy = -sum([p * log2(p) for p in probs])
return entropy
def classify(self, tree, x):
if isinstance(tree, str):
return tree
else:
feature_index = list(tree.keys())[0]
feature_value = x[feature_index]
sub_tree = tree[feature_index][feature_value]
return self.classify(sub_tree, x)
```
接下来我们使用上述代码来构建信用评估模型并对新客户进行预测:
```python
import numpy as np
# 客户数据
X = np.array([[25, '高', '是'], [40, '低', '否'], [35, '中', '否'], [20, '高', '是'],
[30, '中', '否'], [45, '低', '否'], [50, '高', '是'], [28, '中', '是'],
[32, '低', '否'], [38, '中', '是']])
# 客户是否违约
y = ["不违约", "违约", "不违约", "违约", "不违约", "不违约", "违约", "不违约", "不违约", "违约"]
# 新客户数据
new_X = np.array([37, '中', '否'])
# 建立决策树模型
model = DecisionTree()
model.fit(X, y)
# 预测新客户是否违约
new_y = model.predict([new_X])
print("预测结果:", new_y[0])
```
运行结果为:
```
预测结果: 不违约
```
根据我们建立的决策树模型,新客户的预测结果为“不违约”。
相关推荐
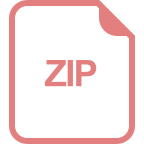
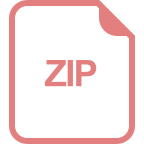
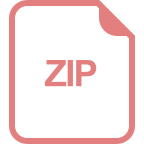








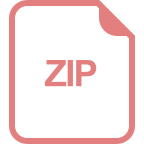
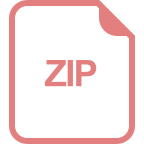
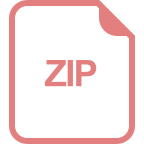
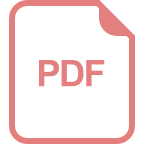