springboot注册bean的注解
时间: 2023-05-03 07:00:22 浏览: 57
SpringBoot中的注解@Bean用来声明一个bean,即IoC容器中管理的对象。当IoC容器扫描到被此注解标记的类时,会自动创建一个对象并将其加入到容器中。可以通过@Autowired注解将这个对象注入到其他需要它的地方。同时,@Bean注解也支持传入参数来初始化对象,例如配置参数。
相关问题
Springboot:bean注解注入使用案例
好的,下面是一个简单的 Spring Boot 中使用 `@Autowired` 注解注入 bean 的例子:
1. 首先,我们需要定义一个 bean 类,例如 `UserService`:
```java
@Service
public class UserService {
public void addUser(String username, String password) {
// 添加用户的逻辑
}
}
```
这里使用了 `@Service` 注解来声明 `UserService` 是一个 Spring Bean。
2. 然后,在需要使用 `UserService` 的地方,使用 `@Autowired` 注解将其注入:
```java
@Controller
public class UserController {
@Autowired
private UserService userService;
@PostMapping("/user/add")
public String addUser(@RequestParam("username") String username, @RequestParam("password") String password) {
userService.addUser(username, password);
return "success";
}
}
```
这里使用了 `@Controller` 注解来声明 `UserController` 是一个 Spring MVC 控制器,并使用 `@Autowired` 注解将 `UserService` 注入到 `UserController` 中。
这样,当请求到达 `/user/add` 路径时,`UserController` 的 `addUser` 方法会被调用,其中使用了注入的 `userService` 对象来添加用户。
注意,在使用 `@Autowired` 注入 bean 时,Spring 会根据类型自动查找对应的 bean,如果有多个类型相同的 bean,可以使用 `@Qualifier` 注解指定具体的 bean 名称。
Springboot注册bean方式
Spring Boot提供了多种方式来注册bean:
1. 使用@Component、@Service、@Controller、@Repository注解将类标记为bean,Spring Boot将自动扫描并注册这些bean。
2. 使用@Bean注解手动注册bean,将该注解标注在一个方法上,方法的返回值将被注册为bean。
3. 使用@Configuration注解标记一个类,将@Bean注解标注在类方法上,将该方法返回的对象注册为bean。
4. 使用@Import注解导入一个配置类,该配置类中使用@Bean注解注册bean。
5. 使用@Conditional注解和自定义的条件类来控制bean的注册,当条件满足时才注册bean。
6. 使用@ImportResource注解导入XML配置文件,将其中定义的bean注册到Spring容器中。
7. 使用FactoryBean来创建bean,FactoryBean是一个特殊的bean,它的作用是创建其他bean。在使用FactoryBean时,需要将其注册到Spring容器中。
以上是Spring Boot中注册bean的主要方式,不同的场景和需求可以选择不同的方式。
相关推荐
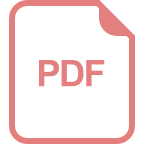
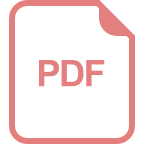
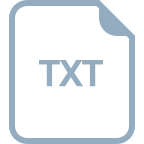












