java gui 动态显示倒计时
时间: 2023-08-11 11:08:36 浏览: 87
要在Java GUI中动态显示倒计时,您可以使用Swing或JavaFX库,并在单独的线程中更新倒计时。下面是一个使用Swing的示例,演示如何在GUI中显示动态倒计时:
```java
import javax.swing.*;
import java.awt.*;
import java.text.SimpleDateFormat;
import java.util.Date;
public class DynamicCountdownGUIExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Dynamic Countdown GUI Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create a label to display the countdown
JLabel countdownLabel = new JLabel();
countdownLabel.setFont(new Font("Arial", Font.BOLD, 48));
frame.add(countdownLabel);
// Calculate the end time of the countdown (10 seconds from now)
Date endTime = new Date(System.currentTimeMillis() + 10000);
// Update the countdown every second
new Thread(() -> {
while (true) {
// Calculate the remaining time
long remainingTime = endTime.getTime() - System.currentTimeMillis();
if (remainingTime <= 0) {
countdownLabel.setText("Time's up!");
break;
}
// Format the remaining time as "mm:ss"
SimpleDateFormat format = new SimpleDateFormat("mm:ss");
String formattedTime = format.format(new Date(remainingTime));
countdownLabel.setText(formattedTime);
// Wait for one second
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}).start();
// Set the size of the frame and show it
frame.setSize(400, 200);
frame.setVisible(true);
}
}
```
在此示例中,我们创建了一个JFrame并向其中添加一个JLabel。我们计算倒计时的结束时间,并使用一个单独的线程来更新倒计时。我们使用SimpleDateFormat格式化倒计时,并将其显示在JLabel中。如果倒计时结束,我们将在JLabel中显示"Time's up!"。使用Thread.sleep方法使线程等待一秒钟,然后再更新倒计时。这样,我们就可以创建一个动态倒计时GUI了。
相关推荐
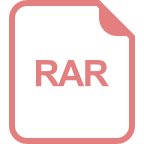
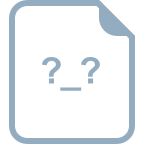
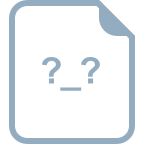














