三层架构学生管理系统简单代码
时间: 2023-07-28 17:07:40 浏览: 41
以下是一个简单的三层架构学生管理系统的代码示例:
**数据访问层(Data Access Layer,DAL):**
```csharp
public class StudentDAL
{
private SqlConnection connection;
public StudentDAL(string connectionString)
{
connection = new SqlConnection(connectionString);
}
public List<Student> GetStudents()
{
List<Student> students = new List<Student>();
SqlCommand command = new SqlCommand("SELECT * FROM Students", connection);
connection.Open();
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
Student student = new Student();
student.ID = Convert.ToInt32(reader["ID"]);
student.Name = reader["Name"].ToString();
student.Age = Convert.ToInt32(reader["Age"]);
students.Add(student);
}
reader.Close();
connection.Close();
return students;
}
public void AddStudent(Student student)
{
SqlCommand command = new SqlCommand("INSERT INTO Students (Name, Age) VALUES (@Name, @Age)", connection);
command.Parameters.AddWithValue("@Name", student.Name);
command.Parameters.AddWithValue("@Age", student.Age);
connection.Open();
command.ExecuteNonQuery();
connection.Close();
}
}
```
**业务逻辑层(Business Logic Layer,BLL):**
```csharp
public class StudentBLL
{
private StudentDAL studentDAL;
public StudentBLL(string connectionString)
{
studentDAL = new StudentDAL(connectionString);
}
public List<Student> GetStudents()
{
return studentDAL.GetStudents();
}
public void AddStudent(Student student)
{
studentDAL.AddStudent(student);
}
}
```
**用户界面层(User Interface Layer,UI):**
```csharp
public partial class MainForm : Form
{
private StudentBLL studentBLL;
public MainForm()
{
InitializeComponent();
studentBLL = new StudentBLL("connectionString");
}
private void MainForm_Load(object sender, EventArgs e)
{
RefreshStudentList();
}
private void btnAdd_Click(object sender, EventArgs e)
{
Student student = new Student();
student.Name = txtName.Text;
student.Age = Convert.ToInt32(txtAge.Text);
studentBLL.AddStudent(student);
RefreshStudentList();
}
private void RefreshStudentList()
{
List<Student> students = studentBLL.GetStudents();
lvStudents.Items.Clear();
foreach (Student student in students)
{
ListViewItem item = new ListViewItem(student.ID.ToString());
item.SubItems.Add(student.Name);
item.SubItems.Add(student.Age.ToString());
lvStudents.Items.Add(item);
}
}
}
```
以上代码示例中,数据访问层负责与数据库交互,业务逻辑层负责处理业务逻辑,用户界面层负责与用户进行交互。三层架构可以有效地分离关注点,使得代码更加清晰、易于维护。
相关推荐
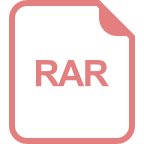
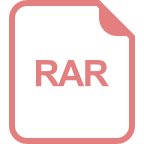
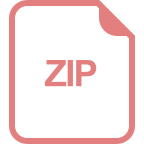














