mapbox-gl-draw 绘制箭头控件
时间: 2023-10-26 09:05:41 浏览: 60
Mapbox-GL-Draw是一款基于Mapbox GL JS的开源绘图库。它提供了一系列的绘图工具,可以在地图上绘制点、线、面等要素。同时,它也支持自定义绘图控件,可以满足不同的绘图需求。
要绘制箭头控件,可以使用Mapbox-GL-Draw的自定义绘图控件功能。具体步骤如下:
1. 创建一个绘图控件,继承自`mapboxgl.Draw`:
```javascript
var ArrowControl = mapboxgl.Draw.extend({
// ...
});
```
2. 在控件的`onAdd`方法中创建绘图工具,并添加箭头绘制模式:
```javascript
ArrowControl.prototype.onAdd = function(map) {
// ...
this.addMode('arrow', ArrowMode);
// ...
}
```
3. 定义箭头绘制模式:
```javascript
var ArrowMode = {
// ...
onSetup: function() {
// ...
},
onDrag: function() {
// ...
},
onClick: function() {
// ...
},
// ...
};
```
4. 在`onSetup`方法中创建箭头要素,并添加到地图上:
```javascript
onSetup: function() {
var arrow = {
type: 'Feature',
geometry: {
type: 'LineString',
coordinates: []
},
properties: {
'arrow': 'true'
}
};
this.addFeature(arrow);
this.arrow = arrow;
// ...
},
```
5. 在`onDrag`方法中更新箭头要素的坐标:
```javascript
onDrag: function(event) {
var arrow = this.arrow;
var coords = arrow.geometry.coordinates;
if (coords.length === 0) {
coords.push(event.lngLat.toArray());
coords.push(event.lngLat.toArray());
this.updateUIClasses({mouse: 'add'});
} else {
coords[1] = event.lngLat.toArray();
this.updateUIClasses({mouse: 'mousemove'});
}
arrow.geometry.coordinates = coords;
this.map.fire('draw.update', {action: 'change', feature: arrow});
},
```
6. 在`onClick`方法中结束绘制:
```javascript
onClick: function(event) {
var arrow = this.arrow;
var coords = arrow.geometry.coordinates;
if (coords.length === 2) {
this.updateUIClasses({mouse: 'pointer'});
this.changeMode('simple_select', {featureIds: [arrow.id]});
}
},
```
7. 最后,在地图上添加箭头控件:
```javascript
map.addControl(new ArrowControl());
```
以上就是绘制箭头控件的基本流程,具体实现可以参考Mapbox-GL-Draw的官方文档。
相关推荐
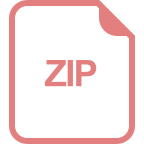
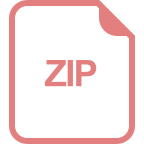
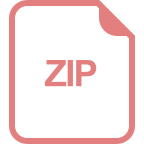














