写一段代码,运用jsp,实现“评教”功能,给教师打分,分数存储到数据库,要求前端页面能够显示数据库已有的教师姓名,且实现打分和提交功能
时间: 2024-03-27 19:41:09 浏览: 63
以下是一个简单的 JSP 代码示例,实现评教功能并将分数存储到数据库中:
评教页面(index.jsp):
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>评教</title>
</head>
<body>
<h1>教师评分</h1>
<form action="submit.jsp" method="post">
<label for="teacher">选择教师:</label>
<select name="teacher" id="teacher">
<!-- 动态生成教师列表 -->
<%
// 假设教师列表存储在名为 teachers 的 List 对象中
List<String> teachers = new ArrayList<String>();
teachers.add("张三");
teachers.add("李四");
teachers.add("王五");
for (String teacher : teachers) {
out.println("<option value=\"" + teacher + "\">" + teacher + "</option>");
}
%>
</select>
<br>
<label for="score">评分:</label>
<input type="number" name="score" id="score" min="0" max="100" required>
<br>
<input type="submit" value="提交">
</form>
</body>
</html>
```
提交评分数据(submit.jsp):
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>提交评分</title>
</head>
<body>
<%
// 获取评分数据
String teacher = request.getParameter("teacher");
int score = Integer.parseInt(request.getParameter("score"));
// 连接数据库
String url = "jdbc:mysql://localhost:3306/test?useSSL=false&serverTimezone=UTC";
String username = "root";
String password = "password";
Connection con = DriverManager.getConnection(url, username, password);
// 插入评分数据
String sql = "INSERT INTO scores (teacher, score) VALUES (?, ?)";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setString(1, teacher);
pstmt.setInt(2, score);
pstmt.executeUpdate();
// 关闭数据库连接
pstmt.close();
con.close();
%>
<h1>提交成功</h1>
<p>您对 <%= teacher %> 的评分为 <%= score %>。</p>
</body>
</html>
```
说明:
本示例中,假设教师列表存储在名为 teachers 的 List 对象中,然后使用 JSP 动态生成教师下拉列表。在提交评分数据时,使用 JDBC 连接 MySQL 数据库,将评分数据插入名为 scores 的表中。需要注意的是,示例中的数据库连接信息(url、username、password)需要根据实际情况修改。
阅读全文
相关推荐








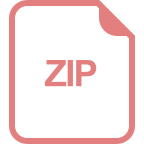
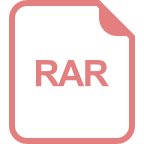
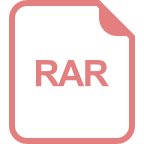


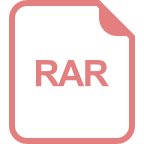




