PNormalLineEdit=QLineEdit() PButtonSearch=QPushButton('查询已有') PButtonInsert=QPushButton('插入上述')
时间: 2024-03-12 09:43:41 浏览: 14
这是 PyQt5 中创建小部件的代码。在这个例子中,我们创建了三个小部件:一个文本框和两个按钮。具体的代码如下:
```python
PNormalLineEdit=QLineEdit() # 创建一个文本框
PButtonSearch=QPushButton('查询已有') # 创建一个按钮,按钮上显示“查询已有”
PButtonInsert=QPushButton('插入上述') # 创建一个按钮,按钮上显示“插入上述”
```
`QLineEdit` 是一个简单的文本框控件,它可以用于输入和显示单行文本。在这个例子中,我们创建了一个名为 `PNormalLineEdit` 的文本框控件。
`QPushButton` 是一个常用的按钮控件,它可以用于触发事件和执行操作。在这个例子中,我们创建了两个按钮控件:`PButtonSearch` 和 `PButtonInsert`。
在创建小部件之后,我们可以将它们添加到布局中,以便在界面中显示。例如,我们可以使用 `QFormLayout` 布局类将文本框和按钮组合成一个表单,并将它们添加到主窗口中。通过使用小部件和布局类,我们可以轻松地创建复杂的用户界面,并使其看起来更加美观和易于使用。
相关问题
pyqt5 QLineEdit 如何插入Qpushbutton控件
在 PyQt5 中,可以使用 QLineEdit 的 setButton() 方法来插入 QPushButton 控件。具体步骤如下:
1. 创建 QLineEdit 控件和 QPushButton 控件:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QLineEdit, QPushButton
app = QApplication([])
window = QWidget()
lineEdit = QLineEdit()
pushButton = QPushButton("Button")
window.show()
app.exec_()
```
2. 在 QLineEdit 控件中插入 QPushButton 控件:
```python
lineEdit.setButton(0, pushButton)
```
其中,第一个参数表示插入的按钮的位置,0 表示在 QLineEdit 控件的左侧插入按钮;第二个参数为 QPushButton 控件。
完整代码如下:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QLineEdit, QPushButton
app = QApplication([])
window = QWidget()
lineEdit = QLineEdit()
pushButton = QPushButton("Button")
lineEdit.setButton(0, pushButton)
window.show()
app.exec_()
```
使用c++如何将QLineEdit控件和一个QPushButton控件连接
可以使用Qt的信号与槽机制,将QLineEdit控件的textChanged信号连接到QPushButton控件的clicked信号,实现当QLineEdit控件文本发生改变时,点击QPushButton控件的响应。
下面是示例代码:
```
#include <QApplication>
#include <QLineEdit>
#include <QPushButton>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QLineEdit *lineEdit = new QLineEdit();
QPushButton *pushButton = new QPushButton("Click me");
QObject::connect(lineEdit, &QLineEdit::textChanged, pushButton, &QPushButton::clicked);
lineEdit->show();
pushButton->show();
return app.exec();
}
```
在上述代码中,我们使用QObject::connect()方法将QLineEdit控件的textChanged信号连接到QPushButton控件的clicked信号,实现了二者的联动。通过这种机制,当QLineEdit控件的文本改变时,就会触发QPushButton控件的点击事件。
相关推荐
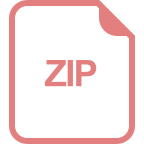
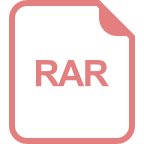
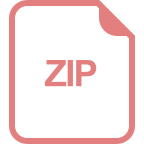













