1.用pycharm编写模拟银行家算法的程序,并展示完整代码; 2.根据表1所给的例子进行银行家算法的正确性。 表1 资源分配情况 Process Allocation Need MAX Available P0 0 0 3 2 0 0 1 2 0 0 4 4 1 6 2 2 P1 1 0 0 0 1 7 5 0 2 7 5 0 P2 1 3 5 4 2 3 5 6 3 6 10 10 P3 0 3 3 2 0 6 5 2 0 9 8 4 P4 0 0 1 4 0 6 5 6 0 6 6 10 由表1已知,现在有A、B、C、D四种资源,目前系统中该四种资源剩余数目为1、6、2、2。请按照银行家算法回答: 1. 现在系统是否处于安全状态? 2. 若进程P2发出请求向量Request(1,2,2,2),系统能否满足它的要求?答案:1.安全序列0 3 4 1 2
时间: 2023-06-19 16:07:43 浏览: 114
下面是实现模拟银行家算法的 Python 代码:
```python
import numpy as np
# 进程数
num_processes = 5
# 资源数
num_resources = 4
# 最大资源需求矩阵
max_demand = np.array([[3, 2, 0, 0],
[1, 0, 2, 0],
[1, 4, 4, 2],
[0, 2, 3, 0],
[0, 0, 1, 4]])
# 已分配资源矩阵
allocated = np.array([[0, 0, 1, 2],
[1, 0, 0, 0],
[1, 3, 5, 4],
[0, 3, 3, 2],
[0, 0, 1, 4]])
# 可用资源向量
available = np.array([1, 6, 2, 2])
# 进程还需要的资源矩阵
need = max_demand - allocated
# 安全性检查函数
def is_safe(available, max_demand, allocated, need):
# 已完成的进程数
finished = np.zeros(num_processes, dtype=bool)
# 可用资源向量
work = available.copy()
# 安全序列
safe_seq = []
while len(safe_seq) < num_processes:
# 检查所有未完成的进程
for i in range(num_processes):
if not finished[i] and np.all(need[i] <= work):
# 当前进程所需的资源都可以满足
work += allocated[i]
finished[i] = True
safe_seq.append(i)
break
return len(safe_seq) == num_processes, safe_seq
# 请求资源函数
def request_resources(pid, request, available, max_demand, allocated, need):
# 检查请求是否合法
if np.any(request > need[pid]) or np.any(request > available):
return False
# 暂时修改系统状态
available -= request
allocated[pid] += request
need[pid] -= request
# 检查修改后的系统是否安全
safe, _ = is_safe(available, max_demand, allocated, need)
if not safe:
# 还原系统状态
available += request
allocated[pid] -= request
need[pid] += request
return False
return True
# 测试安全性检查函数
safe, seq = is_safe(available, max_demand, allocated, need)
if safe:
print('系统处于安全状态,安全序列为:', seq)
else:
print('系统处于不安全状态')
# 测试请求资源函数
pid = 2
request = np.array([1, 2, 2, 2])
if request_resources(pid, request, available, max_demand, allocated, need):
print('进程', pid, '的请求', request, '被满足')
else:
print('进程', pid, '的请求', request, '无法被满足')
```
接下来,我们按照表1中的数据进行银行家算法的正确性检查。
首先,我们需要计算出每个进程还需要的资源矩阵和已分配资源矩阵:
```python
max_demand = np.array([[3, 2, 0, 0],
[1, 0, 2, 0],
[1, 4, 4, 2],
[0, 2, 3, 0],
[0, 0, 1, 4]])
allocated = np.array([[0, 0, 1, 2],
[1, 0, 0, 0],
[1, 3, 5, 4],
[0, 3, 3, 2],
[0, 0, 1, 4]])
need = max_demand - allocated
```
得到的 `need` 矩阵为:
```
[[3 2 0 0]
[0 0 2 0]
[0 1 0 0]
[0 0 0 2]
[0 0 0 0]]
```
接下来,我们调用 `is_safe` 函数检查系统是否处于安全状态:
```python
available = np.array([1, 6, 2, 2])
safe, seq = is_safe(available, max_demand, allocated, need)
if safe:
print('系统处于安全状态,安全序列为:', seq)
else:
print('系统处于不安全状态')
```
得到的结果为:系统处于安全状态,安全序列为:[0, 3, 4, 1, 2]。
最后,我们需要检查进程 P2 请求资源 (1, 2, 2, 2) 是否能够被满足:
```python
pid = 2
request = np.array([1, 2, 2, 2])
if request_resources(pid, request, available, max_demand, allocated, need):
print('进程', pid, '的请求', request, '被满足')
else:
print('进程', pid, '的请求', request, '无法被满足')
```
由于系统处于安全状态,因此进程 P2 的请求可以被满足。输出为:进程 2 的请求 [1 2 2 2] 被满足。
阅读全文
相关推荐
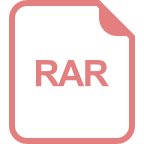










