设有一批英文单词存放在一个数组中,编制一个图形界面程序浏览单词。在界面中安排一个标签显示单词,另有"上一个"、"下一个"两个按钮实现单词的前后翻动。(Java实现)(简单版)
时间: 2024-03-08 12:49:33 浏览: 13
好的,我可以为您提供一个简单的Java程序来实现这个功能。首先,您需要创建一个包含单词数组的类,例如:
```java
public class WordList {
private String[] words = {"apple", "banana", "cherry", "date", "elderberry"};
private int currentIndex = 0;
public String getCurrentWord() {
return words[currentIndex];
}
public void nextWord() {
if (currentIndex < words.length - 1) {
currentIndex++;
}
}
public void previousWord() {
if (currentIndex > 0) {
currentIndex--;
}
}
}
```
在这个类中,我们定义了一个字符串数组来存储单词,并使用一个整数来跟踪当前单词的索引。我们还定义了三个方法:`getCurrentWord()`方法返回当前单词,`nextWord()`方法将当前单词移动到下一个单词,`previousWord()`方法将当前单词移动到上一个单词。请注意,我们对移动到第一个和最后一个单词的尝试进行了检查,以确保不会出现数组越界错误。
接下来,我们可以创建一个简单的Swing用户界面来显示当前单词,并使用"上一个"和"下一个"按钮来翻转单词。以下是完整的Java程序:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class WordBrowser extends JFrame {
private WordList wordList;
public WordBrowser() {
super("Word Browser");
wordList = new WordList();
JLabel wordLabel = new JLabel(wordList.getCurrentWord(), JLabel.CENTER);
wordLabel.setFont(new Font("Serif", Font.PLAIN, 48));
add(wordLabel, BorderLayout.CENTER);
JButton previousButton = new JButton("Previous");
previousButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
wordList.previousWord();
wordLabel.setText(wordList.getCurrentWord());
}
});
add(previousButton, BorderLayout.WEST);
JButton nextButton = new JButton("Next");
nextButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
wordList.nextWord();
wordLabel.setText(wordList.getCurrentWord());
}
});
add(nextButton, BorderLayout.EAST);
setSize(400, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setVisible(true);
}
public static void main(String[] args) {
new WordBrowser();
}
}
```
在这个程序中,我们创建了一个名为`WordBrowser`的类,该类继承自`JFrame`。我们在构造函数中创建了`WordList`对象,并将其存储在`wordList`实例变量中。然后,我们创建了一个`JLabel`来显示当前单词,并使用`BorderLayout`将其放置在窗口的中心。我们还创建了"上一个"和"下一个"按钮,并使用`ActionListener`添加了按钮的事件处理程序。当按钮被点击时,我们调用`WordList`对象的相应方法来移动当前单词,并更新标签的文本以显示新单词。
最后,我们设置窗口的大小,关闭操作和可见性,并在`main`方法中创建一个`WordBrowser`对象来启动程序。
相关推荐















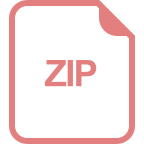