c++移除string 中的空格
时间: 2024-12-15 16:15:47 浏览: 36
在C++中,如果你想要移除`std::string`中的空格,可以使用`std::replace`函数或者遍历整个字符串并跳过空格。以下是两种常见的做法:
1. **使用`std::replace`函数**:
```cpp
std::string str = "your string with spaces";
for (size_t i = 0; i < str.length(); ++i) {
if (str[i] == ' ') {
str.replace(i, 1, "");
}
}
```
这段代码会找到所有的空格并替换为空。
2. **遍历并忽略空格**:
```cpp
std::string str = "your string with spaces";
str.erase(std::remove(str.begin(), str.end(), ' '), str.end());
```
`std::remove`会移动非空格字符到数组前面,然后`erase`会移除所有空格字符的位置。
这两种方法都会得到一个没有空格的新字符串。注意,如果需要处理其他空白字符(如制表符、换行符),可以在比较条件里加入相应的字符。
相关问题
写一个C++函数能移除string中的空格
当然,下面是一个简单的 C++ 函数,它使用了 `erase` 和 `insert` 方法来移除 `std::string` 中的所有空格:
```cpp
#include <string>
std::string removeSpaces(const std::string& input) {
// 创建一个新的字符串,用于存储不包含空格的结果
std::string result;
// 遍历输入字符串,如果当前字符不是空格,则添加到结果中
for (char c : input) {
if (c != ' ') {
result += c;
}
}
return result;
}
```
这个函数接受一个 `const std::string` 类型的引用作为参数,遍历输入字符串,跳过所有空格,并把非空格字符追加到新的结果字符串中。
如果你需要处理大量数据,`std::stringstream` 方法可能会相对高效一些,因为它是按词分割的,但这通常只在你知道如何优化的情况下适用。
c++中删除string里的空格
在C++中,如果你想要删除字符串中的所有空格,你可以使用`std::remove`和`std::replace`函数,这两个函数都属于 `<algorithm>` 库。首先,你需要创建一个迭代器范围,表示要删除的字符位置,然后用一个新的`std::string`来替换原始字符串,并移除指定字符。这里是一个示例:
```cpp
#include <algorithm>
#include <string>
// 原始字符串
std::string str = "Hello World with spaces";
// 创建一个新的迭代器范围,指向所有的空格字符
auto it = std::find_if(str.begin(), str.end(), [](char c) { return std::isspace(c); });
// 如果找到至少一个空格
if (it != str.end()) {
// 找到第一个非空格字符的位置
auto end = std::remove(it, str.end(), ' ');
// 移动结束位置到新的字符串长度
str.erase(end, str.end());
// 如果原字符串中有连续的空格,可以再次查找并移除
while (std::isspace(*str.rbegin())) {
str.pop_back();
}
}
// 输出处理后的字符串
cout << str << '\n';
```
这个示例会将空格从`str`中删除,但不会保留相邻空格。如果你想完全去除所有连续的空格,可以在循环里继续检查。
阅读全文
相关推荐
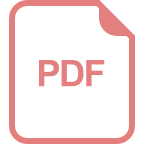
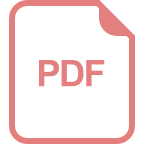
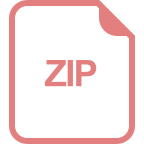

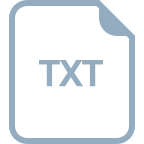











