智能浇花系统Android Studio
时间: 2024-01-10 11:21:33 浏览: 33
智能浇花系统是一种利用Android Studio开发的应用程序,它结合了STM32系列单片机、DTH11温湿度传感器和HC-05蓝牙模块,实现了远程可控的浇花功能。该系统包括一个APK软件和一个智能浇花硬件,可以实时获取温湿度信息并进行短距离控制。
在Android Studio中开发智能浇花系统,你可以使用Java语言编写应用程序的前端界面和逻辑代码。你可以使用Android Studio提供的各种工具和库来创建用户界面、处理用户输入、与蓝牙模块进行通信等。
以下是一个简单的示例代码,演示如何在Android Studio中实现智能浇花系统的功能:
```java
// 导入所需的库
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.bluetooth.BluetoothSocket;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.UUID;
public class MainActivity extends AppCompatActivity {
// 定义蓝牙相关的变量
private BluetoothAdapter bluetoothAdapter;
private BluetoothDevice bluetoothDevice;
private BluetoothSocket bluetoothSocket;
private InputStream inputStream;
private OutputStream outputStream;
private static final UUID MY_UUID = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
// 定义界面上的控件
private TextView temperatureTextView;
private TextView humidityTextView;
private Button waterButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化界面上的控件
temperatureTextView = findViewById(R.id.temperatureTextView);
humidityTextView = findViewById(R.id.humidityTextView);
waterButton = findViewById(R.id.waterButton);
// 初始化蓝牙适配器
bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
// 设置按钮的点击事件
waterButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 发送浇花指令到硬件
try {
outputStream.write("water".getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
});
}
@Override
protected void onResume() {
super.onResume();
// 连接蓝牙设备
bluetoothDevice = bluetoothAdapter.getRemoteDevice("设备的MAC地址");
try {
bluetoothSocket = bluetoothDevice.createRfcommSocketToServiceRecord(MY_UUID);
bluetoothSocket.connect();
inputStream = bluetoothSocket.getInputStream();
outputStream = bluetoothSocket.getOutputStream();
} catch (IOException e) {
e.printStackTrace();
}
// 开始接收温湿度数据
new Thread(new Runnable() {
@Override
public void run() {
byte[] buffer = new byte[1024];
int bytes;
while (true) {
try {
bytes = inputStream.read(buffer);
String data = new String(buffer, 0, bytes);
// 解析温湿度数据并更新界面
String[] values = data.split(",");
final String temperature = values[0];
final String humidity = values[1];
runOnUiThread(new Runnable() {
@Override
public void run() {
temperatureTextView.setText(temperature);
humidityTextView.setText(humidity);
}
});
} catch (IOException e) {
e.printStackTrace();
break;
}
}
}
}).start();
}
@Override
protected void onPause() {
super.onPause();
// 断开蓝牙连接
try {
inputStream.close();
outputStream.close();
bluetoothSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这是一个简单的示例代码,它演示了如何在Android Studio中使用Java语言开发智能浇花系统的应用程序。你可以根据自己的需求进行修改和扩展。
相关推荐
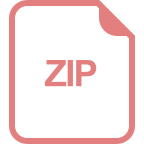














