优化 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd"); Date today = formatter.parse(formatter.format(new Date()));
时间: 2024-04-10 14:33:36 浏览: 139
优化后的代码如下所示:
```java
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
LocalDate today = LocalDate.now();
String todayStr = today.format(formatter);
```
优化的改进点:
1. 使用了Java 8中的新日期时间API(java.time包),而不是旧的SimpleDateFormat类。
2. 使用DateTimeFormatter替代了SimpleDateFormat,提供了更好的线程安全性和易读性。
3. 使用LocalDate.now()获取当前日期,而不是通过new Date()创建一个Date对象。
4. 使用DateTimeFormatter的format方法将LocalDate对象格式化为字符串类型的日期。
通过以上优化,代码更加简洁、可读性更高,并且使用了更现代化的日期时间处理方法。如果需要将日期转换为Date对象,可以使用LocalDate对象的atStartOfDay方法将其转换为LocalDateTime对象,再通过LocalDateTime对象的toInstant方法转换为Date对象。
相关问题
优化SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd"); String today = format.format(new Date()); Date todayDate=format.parse(today);
优化后的代码如下所示:
```java
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
String today = LocalDate.now().format(formatter);
LocalDate todayDate = LocalDate.parse(today, formatter);
```
优化的改进点:
1. 使用了Java 8中的新日期时间API(java.time包),而不是旧的SimpleDateFormat类。
2. 使用DateTimeFormatter替代了SimpleDateFormat,提供了更好的线程安全性和易读性。
3. 使用LocalDate.now()获取当前日期,而不是通过new Date()创建一个Date对象,再格式化为字符串。
4. 使用DateTimeFormatter的parse方法将字符串类型的日期解析为LocalDate对象,而不是使用旧的SimpleDateFormat的parse方法。
通过以上优化,代码更加简洁、可读性更高,并且使用了更现代化的日期时间处理方法。
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
这是Java中用于格式化日期时间的类SimpleDateFormat的创建方式。它表示日期时间的格式为"年-月-日 时:分:秒",可以根据需要修改格式字符串来满足不同的需求。例如,如果要表示毫秒数,则可以在格式字符串中添加"SSS"。使用这个类可以将Date对象格式化为字符串,也可以将字符串解析为Date对象。例如:
```java
Date now = new Date();
String formattedDate = formatter.format(now);
System.out.println("当前时间为:" + formattedDate);
String dateString = "2021-08-01 13:30:00";
Date parsedDate = formatter.parse(dateString);
System.out.println("解析后的日期为:" + parsedDate);
```
输出结果为:
```
当前时间为:2021-08-01 13:30:00
解析后的日期为:Sun Aug 01 13:30:00 CST 2021
```
需要注意的是,SimpleDateFormat是非线程安全的,如果需要在多线程环境下使用,需要采取措施保证线程安全。
阅读全文
相关推荐
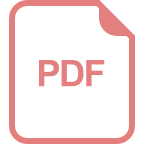













