VS2019 C++ 如何检查文件目录已经存在,如何处理。请用代码说明
时间: 2024-10-20 19:11:28 浏览: 24
在Visual Studio 2019中编写C++程序时,你可以使用标准库中的`std::filesystem`模块来检查文件或目录是否已存在。下面是一个简单的例子:
```cpp
#include <iostream>
#include <filesystem>
namespace fs = std::filesystem;
bool directory_exists(const std::string& path) {
return fs::exists(path) && fs::is_directory(path);
}
bool file_exists(const std::string& path) {
return fs::exists(path) && !fs::is_directory(path);
}
void handle_directory_create(const std::string& path, bool create_if_not_exist = true) {
if (create_if_not_exist && !directory_exists(path)) {
try {
fs::create_directories(path); // 如果不存在则尝试创建
std::cout << "Directory created: " << path << std::endl;
} catch (const fs::filesystem_error& e) {
std::cerr << "Error creating directory: " << e.what() << std::endl;
}
} else if (!create_if_not_exist && !directory_exists(path)) {
std::cerr << "Directory does not exist and creation is disabled: " << path << std::endl;
} else {
std::cout << "Directory exists: " << path << std::endl;
}
}
int main() {
std::string dir_path = "path_to_your_directory";
if (file_exists(dir_path)) {
std::cout << "File exists: " << dir_path << std::endl;
} else {
handle_directory_create(dir_path);
}
return 0;
}
```
在这个示例中,我们首先检查了路径是否存在以及它是否是一个目录。如果需要创建目录,并且目录不存在,我们会尝试使用`fs::create_directories`函数创建。如果没有权限或者发生错误,会捕获异常并打印错误信息。
阅读全文
相关推荐
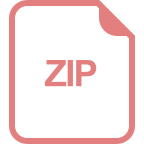
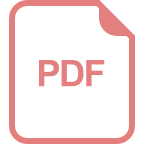
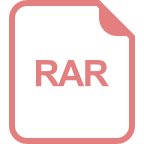

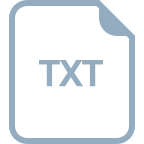
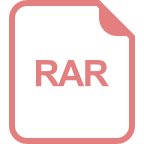
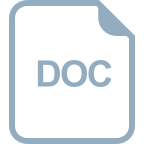
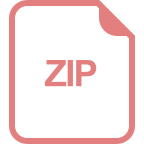
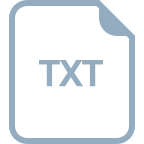
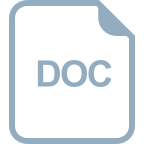
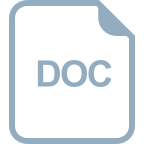
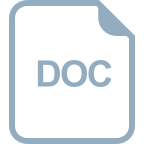
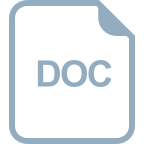
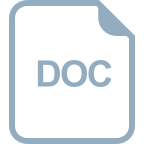
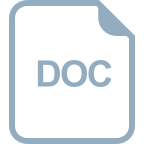
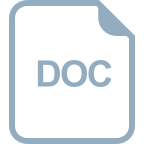
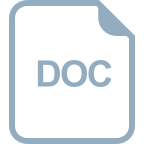
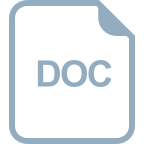
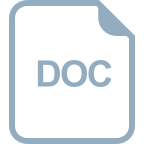