vue3 父子组件传数组
时间: 2024-09-13 07:13:48 浏览: 126
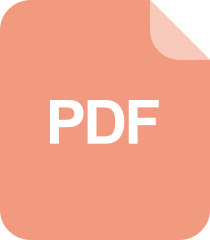
解决Vue中 父子传值 数据丢失问题
Vue 3中父子组件之间传递数组数据,可以通过props和事件来实现。以下是一个基本的示例:
在父组件中,你需要将数组作为prop传递给子组件。假设父组件中有一个名为`items`的数组,你可以这样传递:
```vue
<template>
<ChildComponent :items="items" />
</template>
<script>
import { ref } from 'vue';
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
setup() {
const items = ref(['apple', 'banana', 'cherry']);
return { items };
}
};
</script>
```
在子组件中,你可以接收这个prop并使用它:
```vue
<template>
<ul>
<li v-for="(item, index) in items" :key="index">{{ item }}</li>
</ul>
</template>
<script>
export default {
props: {
items: Array
}
};
</script>
```
如果子组件需要修改这个数组,并且希望父组件能够响应这些变化,你可以使用自定义事件来实现。子组件可以发射一个事件来通知父组件进行数组更新:
```vue
<template>
<button @click="addItem">Add Item</button>
</template>
<script>
export default {
props: {
items: Array
},
methods: {
addItem() {
this.$emit('update:items', [...this.items, 'new-item']);
}
}
};
</script>
```
父组件需要监听这个事件,并更新items:
```vue
<template>
<ChildComponent :items="items" @update:items="handleUpdateItems" />
</template>
<script>
export default {
components: {
ChildComponent
},
setup() {
const items = ref(['apple', 'banana', 'cherry']);
function handleUpdateItems(newItems) {
items.value = newItems;
}
return { items, handleUpdateItems };
}
};
</script>
```
这样,当子组件调用`addItem`方法时,它会通过自定义事件通知父组件,父组件随后会更新其items数组。
阅读全文
相关推荐
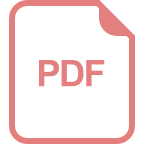
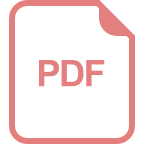






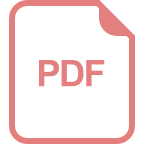








