用java代码文件流怎么实现上传文件到Moonshot Ai
时间: 2024-09-10 19:21:14 浏览: 102
要使用Java代码中的文件流实现上传文件到Moonshot Ai(假设是一个提供API接口的服务),你需要执行以下步骤:
1. 引入必要的库:通常上传文件需要使用`java.net.URLConnection`或`org.apache.http.client.methods.HttpPost`等类,以及处理HTTP请求和响应的库(如Apache HttpClient)。
2. 创建URL连接:根据Moonshot Ai提供的API文档,构造正确的URL和可能需要的参数。
3. 设置请求属性:如果是通过HTTP POST方法上传,需要设置请求头中的`Content-Type`为`multipart/form-data`。同时,如果API需要认证,需要添加相应的认证信息。
4. 读取文件并发送请求:打开文件输入流,读取文件内容,并将文件作为请求的一部分发送到服务器。
下面是一个简化的示例代码,展示了如何使用Java的URLConnection类上传文件:
```java
import java.io.*;
import java.net.*;
public class FileUploader {
public static void main(String[] args) {
String targetURL = "https://api.moonshotai.com/upload"; // Moonshot Ai的上传接口URL
String fileNameToUpload = "example.txt"; // 要上传的文件名
String uploadFilePath = "C:/path/to/your/file/example.txt"; // 文件在本地的路径
try {
// 创建URL对象
URL url = new URL(targetURL);
// 打开连接
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
// 设置请求方法为POST
conn.setRequestMethod("POST");
// 设置请求头,特别是Content-Type
conn.setRequestProperty("Content-Type", "multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW");
// 允许输出
conn.setDoOutput(true);
// 写入请求体数据
try (DataOutputStream out = new DataOutputStream(conn.getOutputStream())) {
// 分割符
String boundary = "----WebKitFormBoundary7MA4YWxkTrZu0gW";
out.writeBytes("--" + boundary + "\r\n");
out.writeBytes("Content-Disposition: form-data; name=\"file\"; filename=\"" + fileNameToUpload + "\"\r\n");
out.writeBytes("Content-Type: " + HttpURLConnection.guessContentTypeFromName(fileNameToUpload) + "\r\n\r\n");
// 读取文件
FileInputStream fileInputStream = new FileInputStream(new File(uploadFilePath));
int bytesAvailable = fileInputStream.available();
int bufferSize = Math.min(bytesAvailable, 1 * 1024 * 1024);
byte[] buffer = new byte[bufferSize];
int bytesRead = fileInputStream.read(buffer, 0, bufferSize);
while (bytesRead > 0) {
out.write(buffer, 0, bufferSize);
bytesAvailable = fileInputStream.available();
bufferSize = Math.min(bytesAvailable, 1 * 1024 * 1024);
bytesRead = fileInputStream.read(buffer, 0, bufferSize);
}
// 添加最后一个分隔符
out.writeBytes("\r\n--" + boundary + "--\r\n");
out.flush();
// 获取服务器响应码
int responseCode = conn.getResponseCode();
System.out.println("Response Code : " + responseCode);
// 根据响应码判断文件是否上传成功
// ...
} catch (IOException e) {
e.printStackTrace();
} finally {
conn.disconnect();
}
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请注意,这个代码示例是基于假设的Moonshot Ai服务和它的API接口。你需要根据实际的API文档来调整URL、请求方法、请求头、参数等信息。
阅读全文
相关推荐
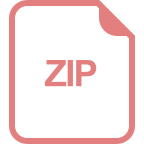
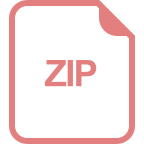
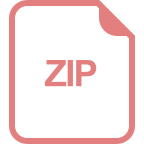
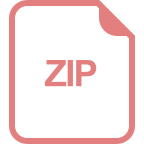
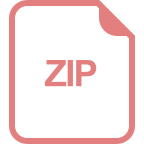
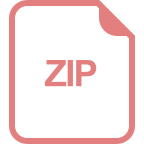
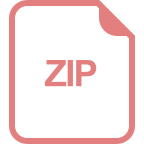

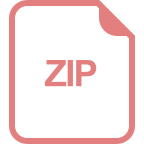
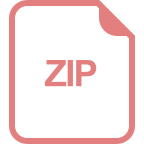
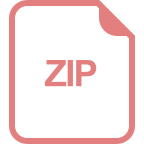
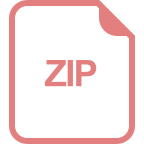
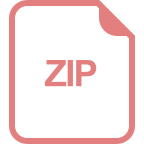
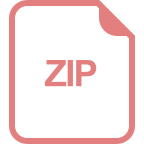
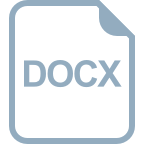
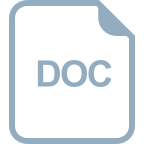
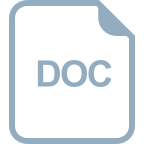

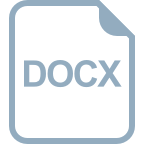