linux中,使用文件IO实现“每隔1秒向文件1.txt写入当前系统时间,行号递增
时间: 2024-10-29 16:13:41 浏览: 23
在Linux中,你可以使用C语言的标准库函数结合定时器来实现这个功能。以下是一个简单的示例,它会在每个秒开始时将当前时间和行号写入`1.txt`文件:
```c
#include <stdio.h>
#include <time.h>
#include <unistd.h> // 包含sleep函数
#define FILE_NAME "1.txt"
#define DELIMITER "\n"
int main() {
FILE *fp;
time_t now;
int line_number = 0;
// 打开文件(如果不存在则创建)
fp = fopen(FILE_NAME, "a+"); // 使用追加模式"a+",避免覆盖已存在的内容
if (fp == NULL) {
perror("Error opening file");
return 1;
}
while (1) {
// 获取当前时间并格式化为字符串
time(&now);
char timestamp[30];
strftime(timestamp, sizeof(timestamp), "%Y-%m-%d %H:%M:%S", localtime(&now));
// 写入时间和行号
fprintf(fp, "%s%04d\n", timestamp, ++line_number);
// 睡眠一秒
sleep(1); // 使用unistd库中的函数
// 如果需要检查退出条件,可以添加以下代码
// 示例:如果达到某个行数阈值就停止
// if (line_number > 100) { // 假设100行之后不再写入
// fclose(fp);
// break;
// }
}
// 关闭文件
fclose(fp);
return 0;
}
```
运行这个程序,`1.txt`文件会每秒钟增加一行,记录当前的时间和对应的行号。
阅读全文
相关推荐
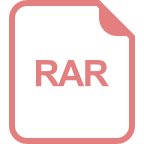
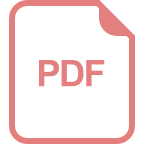
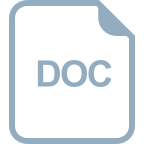






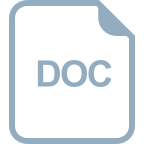
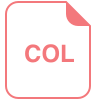

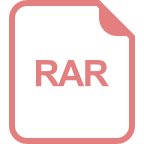
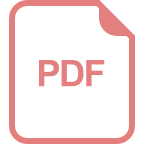
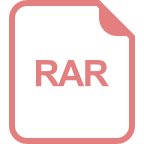
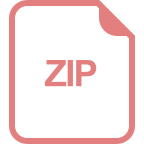
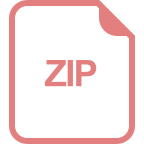