react-native基本语法
时间: 2023-07-29 14:08:34 浏览: 118
React Native是一种用于构建跨平台移动应用的框架,它使用JavaScript和React技术来开发原生移动应用。下面是React Native的一些基本语法:
1. 导入组件:
在使用React Native时,首先需要导入所需的组件。可以使用import语句来导入需要的组件,例如:
```javascript
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
```
2. 创建组件:
使用class关键字或函数来定义一个新的组件。组件必须继承自React.Component类或是函数式组件,例如:
```javascript
class MyComponent extends React.Component {
render() {
return (
<View>
<Text>Hello, World!</Text>
</View>
);
}
}
// 或者是函数式组件
function MyComponent() {
return (
<View>
<Text>Hello, World!</Text>
</View>
);
}
```
3. 渲染组件:
使用ReactDOM.render()方法将组件渲染到指定的DOM元素中,例如:
```javascript
import { AppRegistry } from 'react-native';
AppRegistry.registerComponent('MyApp', () => MyComponent);
```
4. 使用样式:
可以使用StyleSheet.create()方法来创建样式对象,并将其应用于组件的style属性中,例如:
```javascript
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 16,
color: 'blue',
},
});
class MyComponent extends React.Component {
render() {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, World!</Text>
</View>
);
}
}
```
这只是React Native的一些基本语法,还有很多其他的概念和用法,例如组件的生命周期、事件处理等。建议查阅React Native官方文档以获取更详细的信息和示例代码。
阅读全文
相关推荐
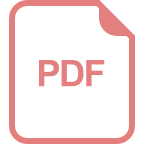
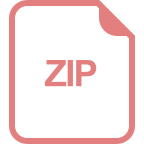
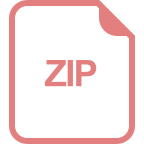
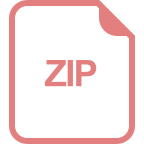
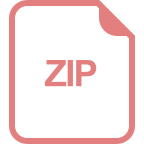
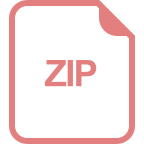
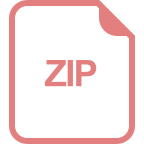
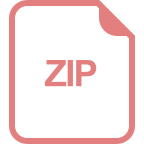
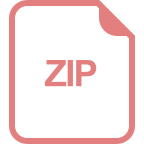
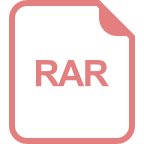
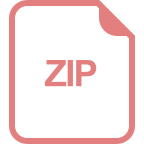
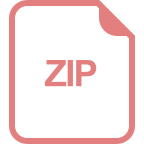
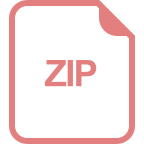
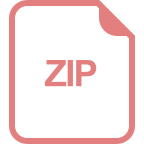
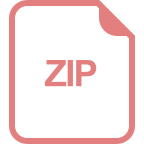
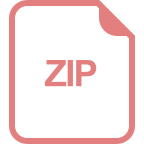
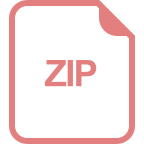