collectors.collectingAndThen
时间: 2024-06-12 12:11:14 浏览: 156
collectors.collectingAndThen是Java 8中的一个收集器,它可以将一个收集器的结果转换为另一种形式。它接受两个参数:一个下游收集器和一个转换函数。它首先使用下游收集器收集元素,然后将结果传递给转换函数进行转换。最终返回转换后的结果。这个方法可以用于对结果进行去重、类型转换、计算等操作。
相关问题
Collectors.collectingAndThen
`Collectors.collectingAndThen` is a static method in the `java.util.stream.Collectors` class that allows you to perform a final transformation on the result of a collector. It takes two arguments: a collector and a `Function` that performs the final transformation.
The `collectingAndThen` method returns a collector that applies the specified collector to the elements of a stream, and then applies the specified function to the result of the collector.
Here's an example of how to use `collectingAndThen` to collect a list of strings, convert it to uppercase, and then return the result as an unmodifiable list:
```
List<String> words = Arrays.asList("hello", "world", "java");
List<String> upperCaseWords = words.stream()
.collect(Collectors.collectingAndThen(
Collectors.toList(),
list -> Collections.unmodifiableList(list.stream()
.map(String::toUpperCase)
.collect(Collectors.toList()))));
System.out.println(upperCaseWords);
```
Output:
```
[HELLO, WORLD, JAVA]
```
In this example, the `collectingAndThen` method first applies the `toList` collector to the stream of strings, which collect them into a mutable `List<String>`. Then, it applies a lambda expression that transforms the list into an unmodifiable list of uppercase strings using the `map` and `collect` methods. Finally, it returns the resulting unmodifiable list.
Java Collectors.collectingAndThen
Java中的Collectors.collectingAndThen方法是一个功能强大的收集器,它可以在一个收集器完成后应用一个函数。这个方法接受两个参数:一个downstream收集器和一个finisher函数。downstream收集器用于执行实际的收集操作,而finisher函数用于对收集结果进行进一步处理。
使用collectingAndThen方法的常见用法是在收集过程完成后,对结果进行转换或处理。例如,如果我们想要将一个List收集器收集到的结果转换为不可变集合,我们可以使用collectingAndThen方法结合toList收集器和ImmutableList的copyOf方法,如下所示:
```java
List<Integer> numbers = Arrays.asList(1, 2, 2, 3, 4, 5);
List<Integer> distinctNumbers = numbers.stream()
.collect(Collectors.collectingAndThen(
Collectors.toList(),
ImmutableList::copyOf
));
```
在这个例子中,我们首先使用toList收集器将流中的元素收集到一个List中。然后,我们使用collectingAndThen方法将这个List作为参数传递给finisher函数ImmutableList::copyOf,将其转换为一个不可变的List。
总结起来,Java的Collectors.collectingAndThen方法可以用于在收集过程完成后对结果进行进一步处理。它接受一个downstream收集器和一个finisher函数作为参数,用于执行收集操作和对结果进行转换或处理。
阅读全文
相关推荐
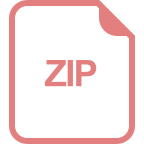
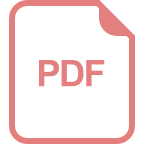













