c#3. 键盘接收n位同学姓名和成绩,输出分数最高的同学的姓名和分数,输出他们的平均分和高于平均分的同学姓名
时间: 2024-10-07 16:04:43 浏览: 23
在C#中,你可以使用`Console.ReadLine()`来获取用户输入的字符串,然后通过字符串分割或者解析为整数来处理姓名和成绩。以下是一个简单的示例程序,它会实现你描述的功能:
```csharp
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("请输入同学姓名和成绩(每行一位同学),以空格隔开,输入'结束'退出:");
string input = "";
double sum = 0;
int count = 0;
Dictionary<string, double> scores = new Dictionary<string, double>();
while ((input = Console.ReadLine()).ToLower() != "结束")
{
if (!string.IsNullOrEmpty(input))
{
string[] nameScore = input.Split(' ');
if (nameScore.Length == 2)
{
string name = nameScore[0];
double score = Convert.ToDouble(nameScore[1]);
// 添加学生姓名和成绩到字典
scores[name] = score;
// 更新总分和人数
sum += score;
count++;
}
}
}
// 计算平均分
double averageScore = count > 0 ? sum / count : 0;
// 输出最高分的学生信息
var maxScoreStudent = scores.OrderByDescending(pair => pair.Value).First();
Console.WriteLine($"分数最高的同学是 {maxScoreStudent.Key},分数是 {maxScoreStudent.Value}。");
// 输出平均分
Console.WriteLine($"平均分为 {averageScore}。");
// 输出高于平均分的学生姓名
foreach (var student in scores)
{
if (student.Value > averageScore)
{
Console.WriteLine($"{student.Key} 的分数高于平均分。");
}
}
Console.ReadLine(); // 等待用户按下Enter键关闭窗口
}
}
```
阅读全文
相关推荐
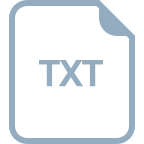
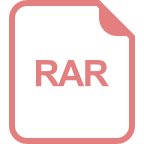







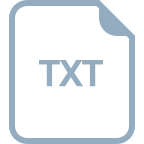
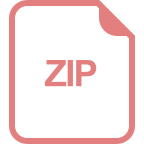
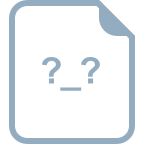