vue3 父组件 调用子组件的方法
时间: 2024-08-15 22:06:12 浏览: 202
在 Vue.js 中,当您想要从父组件调用子组件的方法时,可以通过下面几种方式实现:
### 方式一:直接访问 `$children` 和 `$refs`
#### 示例代码:
假设您有一个名为 `ChildComponent.vue` 的子组件,其中定义了一个方法 `myMethod`。
```html
<!-- ChildComponent.vue -->
<template>
<div>
<!-- ...其他模板内容... -->
<button @click="myMethod">触发子组件方法</button>
</div>
</template>
<script>
export default {
methods: {
myMethod() {
console.log('子组件方法被调用了');
}
}
}
</script>
```
父组件(`App.vue`)可以直接访问 `$children` 或 `$refs` 来调用该方法。
**通过 `$children` 访问:**
```html
<!-- App.vue -->
<template>
<div>
<child-component @my-method="handleMyMethod" />
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
methods: {
handleMyMethod() {
console.log('父组件调用了子组件的方法');
}
}
};
</script>
```
**通过 `$refs` 访问:**
```html
<!-- App.vue -->
<template>
<div>
<child-component ref="childComponent" />
<button @click="callMyMethodFromParent">调用子组件方法</button>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
methods: {
callMyMethodFromParent() {
const childComponent = this.$refs.childComponent;
if (childComponent && childComponent.myMethod) {
childComponent.myMethod();
} else {
console.error('找不到子组件或方法');
}
}
}
};
</script>
```
### 方式二:使用事件总线
如果您不想使用 `$refs` 并希望让应用程序更加模块化和解耦,可以创建一个全局事件总线(Event Bus),用于在不同组件间传递消息。
#### 示例代码:
```javascript
// event-bus.js
const EventBus = new Vue();
export default EventBus;
```
**在父组件中订阅事件并触发子组件方法:**
```html
<!-- App.vue -->
<template>
<div>
<child-component :event-bus="$root.eventBus" />
<button @click="emitMyMethodFromParent">调用子组件方法</button>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
import { eventBus } from '../event-bus.js'; // 引入事件总线
export default {
components: {
ChildComponent,
},
methods: {
emitMyMethodFromParent() {
eventBus.$emit('my-event', '触发子组件方法');
},
onMyMethod(eventData) {
console.log('父组件接收到子组件的消息:', eventData);
}
}
};
</script>
```
**在子组件中监听事件并响应:**
```html
<!-- ChildComponent.vue -->
<template>
<div>
<!-- ...其他模板内容... -->
<button @click="emitMyMethod">触发子组件方法</button>
</div>
</template>
<script>
export default {
mounted() {
this.$root.eventBus.$on('my-event', this.onMyMethod); // 监听事件
},
beforeDestroy() {
this.$root.eventBus.$off('my-event', this.onMyMethod); // 清理监听器
},
methods: {
onMyMethod(eventData) {
console.log('子组件接收到父组件的消息:', eventData);
}
}
}
</script>
```
### 注意事项:
- 当使用 `$refs` 时,确保子组件的方法名和属性名与父组件期望一致,以便能够正确调用。
- 如果方法有返回值,记得在父组件中接收这些返回值。
- 对于更复杂的逻辑或状态管理,考虑使用 Vuex 等状态管理库来提高应用的可维护性。
相关推荐
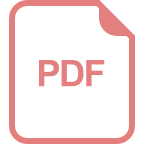















