fold recursive calls
时间: 2024-05-12 08:13:31 浏览: 92
"Folding" recursive calls refers to reducing a recursive function into an iterative one by using a technique called tail recursion. In tail recursion, the recursive call is the last operation performed in a function, allowing the compiler to optimize the call stack and eliminate the need for further recursive calls.
For example, let's say we have a function to calculate the factorial of a number using recursion:
```
int factorial(int n) {
if (n == 0) {
return 1;
}
return n * factorial(n - 1);
}
```
To fold the recursive calls, we can rewrite the function using tail recursion:
```
int factorial_tail(int n, int acc) {
if (n == 0) {
return acc;
}
return factorial_tail(n - 1, n * acc);
}
int factorial(int n) {
return factorial_tail(n, 1);
}
```
In this version, the multiplication is performed before the recursive call, and the result is passed as an accumulator to the next call. This allows the compiler to optimize the function and eliminate the need for a call stack, making it more efficient.
阅读全文
相关推荐
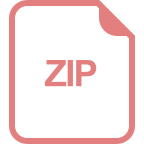
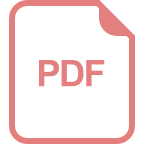
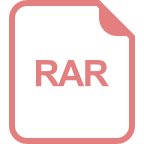
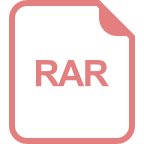
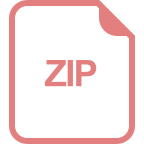
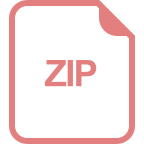
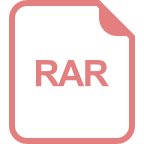







