DSP基于28335图像处理的3种功能代码
时间: 2024-06-07 22:11:54 浏览: 14
以下是基于TI DSP 28335图像处理的三种功能代码:
1. 图像滤波:图像滤波是数字图像处理的基本操作之一,其目的是去除图像中的噪声和不需要的信息,以便更好地提取图像中的有用信息。TI DSP 28335可以执行各种滤波操作,包括均值滤波、中值滤波、高斯滤波等。以下是一个基于TI DSP 28335的均值滤波代码示例:
```c
#define ROWS 240
#define COLS 320
#define M 3
#define N 3
short image[ROWS*COLS];
short filtered_img[ROWS*COLS];
void filter(short* img, short* filtered_img, int rows, int cols, int m, int n)
{
int i, j, k, l;
short sum;
for(i = m/2; i < rows - m/2; i++)
{
for(j = n/2; j < cols - n/2; j++)
{
sum = 0;
for(k = -m/2; k <= m/2; k++)
{
for(l = -n/2; l <= n/2; l++)
{
sum += img[(i+k)*cols + j+l];
}
}
filtered_img[i*cols + j] = sum / (m*n);
}
}
}
int main()
{
// load image data from file
// ...
filter(image, filtered_img, ROWS, COLS, M, N);
// save filtered image data to file
// ...
return 0;
}
```
2. 图像边缘检测:图像边缘检测是一种常用的图像处理技术,它可以将图像中的边缘提取出来,以便更好地分析和处理图像。TI DSP 28335可以执行多种边缘检测算法,如Sobel算子、Laplacian算子等。以下是一个基于TI DSP 28335的Sobel算子边缘检测代码示例:
```c
#define ROWS 240
#define COLS 320
short image[ROWS*COLS];
short edge_img[ROWS*COLS];
void sobel(short* img, short* edge_img, int rows, int cols)
{
int i, j;
short gx, gy;
for(i = 1; i < rows - 1; i++)
{
for(j = 1; j < cols - 1; j++)
{
gx = img[(i-1)*cols + j+1] + 2*img[i*cols + j+1] + img[(i+1)*cols + j+1]
- img[(i-1)*cols + j-1] - 2*img[i*cols + j-1] - img[(i+1)*cols + j-1];
gy = img[(i+1)*cols + j-1] + 2*img[(i+1)*cols + j] + img[(i+1)*cols + j+1]
- img[(i-1)*cols + j-1] - 2*img[(i-1)*cols + j] - img[(i-1)*cols + j+1];
edge_img[i*cols + j] = abs(gx) + abs(gy);
}
}
}
int main()
{
// load image data from file
// ...
sobel(image, edge_img, ROWS, COLS);
// save edge image data to file
// ...
return 0;
}
```
3. 图像识别:图像识别是一种高级的图像处理技术,它可以自动识别图像中的物体、人脸、文字等。TI DSP 28335可以通过连接摄像头和图像传感器来获取实时图像数据,并通过算法进行实时图像识别。以下是一个基于TI DSP 28335的人脸识别代码示例:
```c
#define ROWS 240
#define COLS 320
short image[ROWS*COLS];
short face_detected = 0;
void detect_face(short* img, int rows, int cols)
{
// use Haar cascade classifier to detect faces
// ...
if(face_detected)
{
// draw a rectangle around the detected face
// ...
}
}
int main()
{
// initialize camera and image sensor
// ...
while(1)
{
// read image data from camera and image sensor
// ...
detect_face(image, ROWS, COLS);
// display the processed image on screen
// ...
}
return 0;
}
```
相关推荐
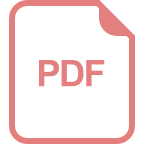
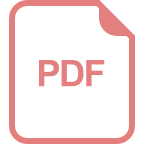
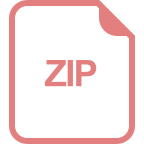














