编写进程互斥与调度的实现代码
时间: 2024-02-18 16:04:36 浏览: 24
下面是一个使用信号量实现进程互斥和调度的示例代码:
```c
#include <stdio.h>
#include <pthread.h>
#include <semaphore.h>
#define THREADS_COUNT 2
sem_t semaphore;
int counter = 0;
void* thread_func(void* thread_id) {
long tid = (long)thread_id;
for (int i = 0; i < 100; i++) {
sem_wait(&semaphore);
counter++;
printf("Thread %ld: counter = %d\n", tid, counter);
sem_post(&semaphore);
}
pthread_exit(NULL);
}
int main() {
pthread_t threads[THREADS_COUNT];
sem_init(&semaphore, 0, 1);
for (long i = 0; i < THREADS_COUNT; i++) {
pthread_create(&threads[i], NULL, thread_func, (void*)i);
}
for (int i = 0; i < THREADS_COUNT; i++) {
pthread_join(threads[i], NULL);
}
sem_destroy(&semaphore);
return 0;
}
```
在此代码中,我们使用了 sem_t 类型的信号量来实现进程互斥和调度。我们创建了两个线程,它们会竞争对 counter 变量的访问。在每个线程的循环中,我们使用 sem_wait() 函数来等待信号量,以确保只有一个线程可以访问 counter 变量;然后,我们使用 sem_post() 函数来释放信号量,以允许其他线程访问 counter 变量。
在主函数中,我们使用 sem_init() 函数来初始化信号量,并使用 pthread_create() 函数来创建两个线程。然后,我们使用 pthread_join() 函数来等待这些线程的完成。最后,我们使用 sem_destroy() 函数来销毁信号量。
当你运行该程序时,你会看到每个线程交替地递增 counter 变量的值,从而实现了进程互斥和调度。
相关推荐
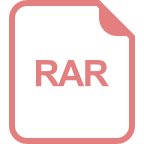
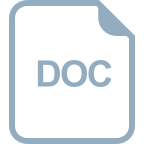
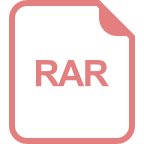














