用Java语言和gui界面实现银行家算法,要求如下(1)进程可动态地申请资源和释放资源,系统按各进程的申请顺序动态地分配资源。 (2)要求程序具有显示和打印各进程的某一时刻的资源分配表和安全序列的功能。 (3)显示和打印各进程依次要求申请的资源号以及为某进程分配资源后的有关资源数据。 (4)可能用到的数据结构: 可利用资源向量Available。它是一个含有m个元素的数组,其中每个元素代表一类可利用资源的数目。 最大需求矩阵Max。nm矩阵,表示n个进程的每一个对m类资源的最大需求。 分配矩阵Allocation 。nm矩阵,表示每个进程已分配的每类资源的数目。 需求矩阵Need 。n*m矩阵,表示每个进程还需要各类资源数。注意代码逻辑通顺,界面清晰明了
时间: 2024-04-08 09:33:58 浏览: 92
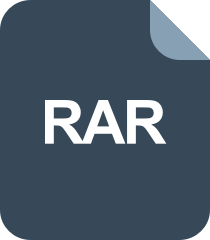
bank.rar_Banker_操作系统_操作系统 银行家算法 java_操作系统银行家算法_银行家算法
下面是一个使用Java语言和GUI界面实现银行家算法的示例代码。代码中使用了Swing来创建GUI界面,实现了您提到的功能要求。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class BankerAlgorithmGUI extends JFrame {
private JLabel[] availableLabels;
private JLabel[][] maxLabels;
private JLabel[][] allocationLabels;
private JLabel[][] needLabels;
private JButton requestButton;
private JTextArea logTextArea;
private int[][] max;
private int[][] allocation;
private int[][] need;
private int[] available;
public BankerAlgorithmGUI() {
super("Banker's Algorithm");
// Initialize the resources
available = new int[]{10, 5, 7};
// Initialize the maximum demand matrix
max = new int[][]{{7, 5, 3}, {3, 2, 2}, {9, 0, 2}, {2, 2, 2}, {4, 3, 3}};
// Initialize the allocation matrix
allocation = new int[][]{{0, 1, 0}, {2, 0, 0}, {3, 0, 2}, {2, 1, 1}, {0, 0, 2}};
// Calculate the need matrix
need = new int[max.length][max[0].length];
for (int i = 0; i < max.length; i++) {
for (int j = 0; j < max[0].length; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
// Create GUI components
JPanel resourcePanel = new JPanel(new GridLayout(1, available.length));
availableLabels = new JLabel[available.length];
for (int i = 0; i < available.length; i++) {
availableLabels[i] = new JLabel("Available: " + available[i]);
resourcePanel.add(availableLabels[i]);
}
JPanel processPanel = new JPanel(new GridLayout(max.length + 1, 1));
maxLabels = new JLabel[max.length][max[0].length];
allocationLabels = new JLabel[max.length][max[0].length];
needLabels = new JLabel[max.length][max[0].length];
for (int i = 0; i < max.length; i++) {
JPanel processRowPanel = new JPanel(new GridLayout(1, 3));
for (int j = 0; j < max[0].length; j++) {
maxLabels[i][j] = new JLabel("Max: " + max[i][j]);
allocationLabels[i][j] = new JLabel("Allocation: " + allocation[i][j]);
needLabels[i][j] = new JLabel("Need: " + need[i][j]);
processRowPanel.add(maxLabels[i][j]);
processRowPanel.add(allocationLabels[i][j]);
processRowPanel.add(needLabels[i][j]);
}
processPanel.add(processRowPanel);
}
requestButton = new JButton("Request Resources");
requestButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// Handle the resource request logic here
// Update the GUI accordingly
}
});
logTextArea = new JTextArea(5, 20);
JScrollPane logScrollPane = new JScrollPane(logTextArea);
// Set up the main content pane
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
contentPane.add(resourcePanel, BorderLayout.NORTH);
contentPane.add(processPanel, BorderLayout.CENTER);
contentPane.add(requestButton, BorderLayout.SOUTH);
contentPane.add(logScrollPane, BorderLayout.EAST);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
BankerAlgorithmGUI gui = new BankerAlgorithmGUI();
gui.setVisible(true);
}
});
}
}
```
请注意,代码中的资源请求逻辑尚未实现。您需要在代码中的 `requestButton` 的 `ActionListener` 中添加您自己的逻辑来处理资源请求,并相应地更新GUI界面。
此示例提供了一个基本的GUI界面,显示了可用资源、最大需求矩阵、分配矩阵和需求矩阵。您可以根据需要进一步完善界面和添加其他功能。
希望这个示例对您有所帮助!如果您有任何疑问,请随时提问。
阅读全文
相关推荐
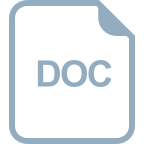
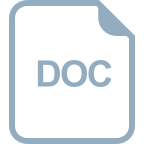


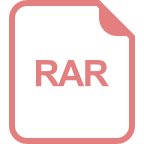
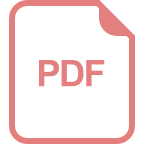
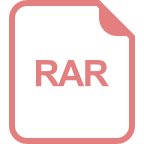
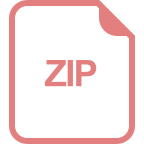
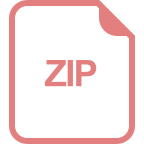
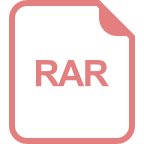
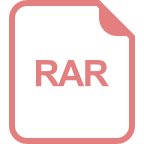
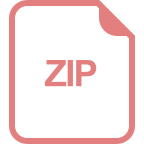
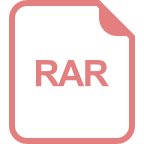
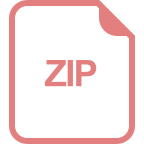
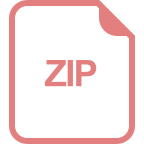
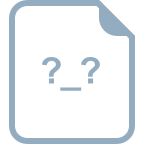
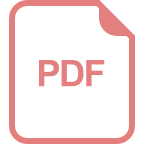
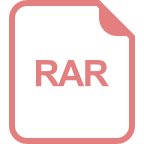